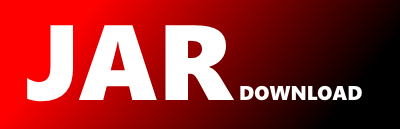
com.pulumi.splunk.GlobalHttpEventCollectorArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.splunk;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GlobalHttpEventCollectorArgs extends com.pulumi.resources.ResourceArgs {
public static final GlobalHttpEventCollectorArgs Empty = new GlobalHttpEventCollectorArgs();
/**
* Number of threads used by HTTP Input server.
*
*/
@Import(name="dedicatedIoThreads")
private @Nullable Output dedicatedIoThreads;
/**
* @return Number of threads used by HTTP Input server.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy