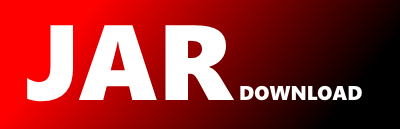
com.pulumi.splunk.OutputsTcpServerArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.splunk;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.splunk.inputs.OutputsTcpServerAclArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OutputsTcpServerArgs extends com.pulumi.resources.ResourceArgs {
public static final OutputsTcpServerArgs Empty = new OutputsTcpServerArgs();
/**
* The app/user context that is the namespace for the resource
*
*/
@Import(name="acl")
private @Nullable Output acl;
/**
* @return The app/user context that is the namespace for the resource
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy