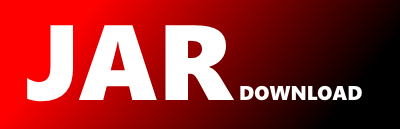
com.pulumi.spotinst.aws.inputs.MrScalarScheduledTaskArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.aws.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MrScalarScheduledTaskArgs extends com.pulumi.resources.ResourceArgs {
public static final MrScalarScheduledTaskArgs Empty = new MrScalarScheduledTaskArgs();
/**
* A cron expression representing the schedule for the task.
*
*/
@Import(name="cron", required=true)
private Output cron;
/**
* @return A cron expression representing the schedule for the task.
*
*/
public Output cron() {
return this.cron;
}
/**
* New desired capacity for the elastigroup.
*
*/
@Import(name="desiredCapacity")
private @Nullable Output desiredCapacity;
/**
* @return New desired capacity for the elastigroup.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy