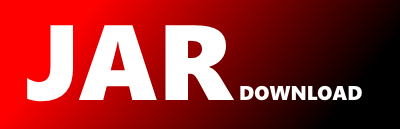
com.pulumi.spotinst.aws.inputs.MrScalarTaskScalingDownPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.aws.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MrScalarTaskScalingDownPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final MrScalarTaskScalingDownPolicyArgs Empty = new MrScalarTaskScalingDownPolicyArgs();
/**
* The type of action to perform. Allowed values are : 'adjustment', 'setMinTarget', 'setMaxTarget', 'updateCapacity', 'percentageAdjustment'
*
*/
@Import(name="actionType")
private @Nullable Output actionType;
/**
* @return The type of action to perform. Allowed values are : 'adjustment', 'setMinTarget', 'setMaxTarget', 'updateCapacity', 'percentageAdjustment'
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy