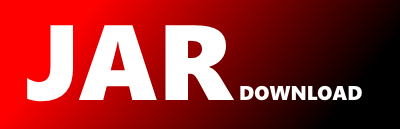
com.pulumi.spotinst.aws.outputs.ElastigroupScalingUpPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.aws.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.spotinst.aws.outputs.ElastigroupScalingUpPolicyDimension;
import com.pulumi.spotinst.aws.outputs.ElastigroupScalingUpPolicyStepAdjustment;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ElastigroupScalingUpPolicy {
/**
* @return The type of action to perform for scaling. Valid values: `"adjustment"`, `"percentageAdjustment"`, `"setMaxTarget"`, `"setMinTarget"`, `"updateCapacity"`. If a `step_adjustment` object is defined, then it cannot be specified.
*
*/
private @Nullable String actionType;
/**
* @return The number of instances to add/remove to/from the target capacity when scale is needed. Can be used as advanced expression for scaling of instances to add/remove to/from the target capacity when scale is needed. You can see more information here: Advanced expression. Example value: `"MAX(currCapacity / 5, value * 10)"`
*
*/
private @Nullable String adjustment;
/**
* @return The amount of time, in seconds, after a scaling activity completes and before the next scaling activity can start. If this parameter is not specified, the default cooldown period for the group applies.
*
*/
private @Nullable Integer cooldown;
/**
* @return A list of dimensions describing qualities of the metric.
*
*/
private @Nullable List dimensions;
/**
* @return The number of periods over which data is compared to the specified threshold.
*
*/
private @Nullable Integer evaluationPeriods;
/**
* @return Specifies whether the scaling policy described in this block is enabled.
*
*/
private @Nullable Boolean isEnabled;
private @Nullable String maxTargetCapacity;
/**
* @return The maximal number of instances to have in the group.
*
*/
private @Nullable String maximum;
/**
* @return The name of the metric, with or without spaces.
*
*/
private String metricName;
/**
* @return . The number of the desired target (and minimum) capacity
*
*/
private @Nullable String minTargetCapacity;
/**
* @return The minimal number of instances to have in the group.
*
*/
private @Nullable String minimum;
/**
* @return The namespace for the alarm's associated metric.
*
*/
private String namespace;
/**
* @return The operator to use in order to determine if the scaling policy is applicable. Valid values: `"gt"`, `"gte"`, `"lt"`, `"lte"`.
*
*/
private @Nullable String operator;
/**
* @return The granularity, in seconds, of the returned datapoints. Period must be at least 60 seconds and must be a multiple of 60.
*
*/
private @Nullable Integer period;
/**
* @return The name of the policy.
*
*/
private String policyName;
/**
* @return The source of the metric. Valid values: `"cloudWatch"`, `"spectrum"`.
*
*/
private @Nullable String source;
/**
* @return The metric statistics to return. For information about specific statistics go to [Statistics](http://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/index.html?CHAP_TerminologyandKeyConcepts.html#Statistic) in the Amazon CloudWatch Developer Guide.
*
*/
private @Nullable String statistic;
private @Nullable List stepAdjustments;
/**
* @return The target number of instances to have in the group.
*
*/
private @Nullable String target;
/**
* @return The value against which the specified statistic is compared. If a `step_adjustment` object is defined, then it cannot be specified.
*
*/
private @Nullable Double threshold;
/**
* @return The unit for the alarm's associated metric. Valid values: `"percent`, `"seconds"`, `"microseconds"`, `"milliseconds"`, `"bytes"`, `"kilobytes"`, `"megabytes"`, `"gigabytes"`, `"terabytes"`, `"bits"`, `"kilobits"`, `"megabits"`, `"gigabits"`, `"terabits"`, `"count"`, `"bytes/second"`, `"kilobytes/second"`, `"megabytes/second"`, `"gigabytes/second"`, `"terabytes/second"`, `"bits/second"`, `"kilobits/second"`, `"megabits/second"`, `"gigabits/second"`, `"terabits/second"`, `"count/second"`, `"none"`.
*
*/
private @Nullable String unit;
private ElastigroupScalingUpPolicy() {}
/**
* @return The type of action to perform for scaling. Valid values: `"adjustment"`, `"percentageAdjustment"`, `"setMaxTarget"`, `"setMinTarget"`, `"updateCapacity"`. If a `step_adjustment` object is defined, then it cannot be specified.
*
*/
public Optional actionType() {
return Optional.ofNullable(this.actionType);
}
/**
* @return The number of instances to add/remove to/from the target capacity when scale is needed. Can be used as advanced expression for scaling of instances to add/remove to/from the target capacity when scale is needed. You can see more information here: Advanced expression. Example value: `"MAX(currCapacity / 5, value * 10)"`
*
*/
public Optional adjustment() {
return Optional.ofNullable(this.adjustment);
}
/**
* @return The amount of time, in seconds, after a scaling activity completes and before the next scaling activity can start. If this parameter is not specified, the default cooldown period for the group applies.
*
*/
public Optional cooldown() {
return Optional.ofNullable(this.cooldown);
}
/**
* @return A list of dimensions describing qualities of the metric.
*
*/
public List dimensions() {
return this.dimensions == null ? List.of() : this.dimensions;
}
/**
* @return The number of periods over which data is compared to the specified threshold.
*
*/
public Optional evaluationPeriods() {
return Optional.ofNullable(this.evaluationPeriods);
}
/**
* @return Specifies whether the scaling policy described in this block is enabled.
*
*/
public Optional isEnabled() {
return Optional.ofNullable(this.isEnabled);
}
public Optional maxTargetCapacity() {
return Optional.ofNullable(this.maxTargetCapacity);
}
/**
* @return The maximal number of instances to have in the group.
*
*/
public Optional maximum() {
return Optional.ofNullable(this.maximum);
}
/**
* @return The name of the metric, with or without spaces.
*
*/
public String metricName() {
return this.metricName;
}
/**
* @return . The number of the desired target (and minimum) capacity
*
*/
public Optional minTargetCapacity() {
return Optional.ofNullable(this.minTargetCapacity);
}
/**
* @return The minimal number of instances to have in the group.
*
*/
public Optional minimum() {
return Optional.ofNullable(this.minimum);
}
/**
* @return The namespace for the alarm's associated metric.
*
*/
public String namespace() {
return this.namespace;
}
/**
* @return The operator to use in order to determine if the scaling policy is applicable. Valid values: `"gt"`, `"gte"`, `"lt"`, `"lte"`.
*
*/
public Optional operator() {
return Optional.ofNullable(this.operator);
}
/**
* @return The granularity, in seconds, of the returned datapoints. Period must be at least 60 seconds and must be a multiple of 60.
*
*/
public Optional period() {
return Optional.ofNullable(this.period);
}
/**
* @return The name of the policy.
*
*/
public String policyName() {
return this.policyName;
}
/**
* @return The source of the metric. Valid values: `"cloudWatch"`, `"spectrum"`.
*
*/
public Optional source() {
return Optional.ofNullable(this.source);
}
/**
* @return The metric statistics to return. For information about specific statistics go to [Statistics](http://docs.aws.amazon.com/AmazonCloudWatch/latest/DeveloperGuide/index.html?CHAP_TerminologyandKeyConcepts.html#Statistic) in the Amazon CloudWatch Developer Guide.
*
*/
public Optional statistic() {
return Optional.ofNullable(this.statistic);
}
public List stepAdjustments() {
return this.stepAdjustments == null ? List.of() : this.stepAdjustments;
}
/**
* @return The target number of instances to have in the group.
*
*/
public Optional target() {
return Optional.ofNullable(this.target);
}
/**
* @return The value against which the specified statistic is compared. If a `step_adjustment` object is defined, then it cannot be specified.
*
*/
public Optional threshold() {
return Optional.ofNullable(this.threshold);
}
/**
* @return The unit for the alarm's associated metric. Valid values: `"percent`, `"seconds"`, `"microseconds"`, `"milliseconds"`, `"bytes"`, `"kilobytes"`, `"megabytes"`, `"gigabytes"`, `"terabytes"`, `"bits"`, `"kilobits"`, `"megabits"`, `"gigabits"`, `"terabits"`, `"count"`, `"bytes/second"`, `"kilobytes/second"`, `"megabytes/second"`, `"gigabytes/second"`, `"terabytes/second"`, `"bits/second"`, `"kilobits/second"`, `"megabits/second"`, `"gigabits/second"`, `"terabits/second"`, `"count/second"`, `"none"`.
*
*/
public Optional unit() {
return Optional.ofNullable(this.unit);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ElastigroupScalingUpPolicy defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String actionType;
private @Nullable String adjustment;
private @Nullable Integer cooldown;
private @Nullable List dimensions;
private @Nullable Integer evaluationPeriods;
private @Nullable Boolean isEnabled;
private @Nullable String maxTargetCapacity;
private @Nullable String maximum;
private String metricName;
private @Nullable String minTargetCapacity;
private @Nullable String minimum;
private String namespace;
private @Nullable String operator;
private @Nullable Integer period;
private String policyName;
private @Nullable String source;
private @Nullable String statistic;
private @Nullable List stepAdjustments;
private @Nullable String target;
private @Nullable Double threshold;
private @Nullable String unit;
public Builder() {}
public Builder(ElastigroupScalingUpPolicy defaults) {
Objects.requireNonNull(defaults);
this.actionType = defaults.actionType;
this.adjustment = defaults.adjustment;
this.cooldown = defaults.cooldown;
this.dimensions = defaults.dimensions;
this.evaluationPeriods = defaults.evaluationPeriods;
this.isEnabled = defaults.isEnabled;
this.maxTargetCapacity = defaults.maxTargetCapacity;
this.maximum = defaults.maximum;
this.metricName = defaults.metricName;
this.minTargetCapacity = defaults.minTargetCapacity;
this.minimum = defaults.minimum;
this.namespace = defaults.namespace;
this.operator = defaults.operator;
this.period = defaults.period;
this.policyName = defaults.policyName;
this.source = defaults.source;
this.statistic = defaults.statistic;
this.stepAdjustments = defaults.stepAdjustments;
this.target = defaults.target;
this.threshold = defaults.threshold;
this.unit = defaults.unit;
}
@CustomType.Setter
public Builder actionType(@Nullable String actionType) {
this.actionType = actionType;
return this;
}
@CustomType.Setter
public Builder adjustment(@Nullable String adjustment) {
this.adjustment = adjustment;
return this;
}
@CustomType.Setter
public Builder cooldown(@Nullable Integer cooldown) {
this.cooldown = cooldown;
return this;
}
@CustomType.Setter
public Builder dimensions(@Nullable List dimensions) {
this.dimensions = dimensions;
return this;
}
public Builder dimensions(ElastigroupScalingUpPolicyDimension... dimensions) {
return dimensions(List.of(dimensions));
}
@CustomType.Setter
public Builder evaluationPeriods(@Nullable Integer evaluationPeriods) {
this.evaluationPeriods = evaluationPeriods;
return this;
}
@CustomType.Setter
public Builder isEnabled(@Nullable Boolean isEnabled) {
this.isEnabled = isEnabled;
return this;
}
@CustomType.Setter
public Builder maxTargetCapacity(@Nullable String maxTargetCapacity) {
this.maxTargetCapacity = maxTargetCapacity;
return this;
}
@CustomType.Setter
public Builder maximum(@Nullable String maximum) {
this.maximum = maximum;
return this;
}
@CustomType.Setter
public Builder metricName(String metricName) {
if (metricName == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "metricName");
}
this.metricName = metricName;
return this;
}
@CustomType.Setter
public Builder minTargetCapacity(@Nullable String minTargetCapacity) {
this.minTargetCapacity = minTargetCapacity;
return this;
}
@CustomType.Setter
public Builder minimum(@Nullable String minimum) {
this.minimum = minimum;
return this;
}
@CustomType.Setter
public Builder namespace(String namespace) {
if (namespace == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "namespace");
}
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder operator(@Nullable String operator) {
this.operator = operator;
return this;
}
@CustomType.Setter
public Builder period(@Nullable Integer period) {
this.period = period;
return this;
}
@CustomType.Setter
public Builder policyName(String policyName) {
if (policyName == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "policyName");
}
this.policyName = policyName;
return this;
}
@CustomType.Setter
public Builder source(@Nullable String source) {
this.source = source;
return this;
}
@CustomType.Setter
public Builder statistic(@Nullable String statistic) {
this.statistic = statistic;
return this;
}
@CustomType.Setter
public Builder stepAdjustments(@Nullable List stepAdjustments) {
this.stepAdjustments = stepAdjustments;
return this;
}
public Builder stepAdjustments(ElastigroupScalingUpPolicyStepAdjustment... stepAdjustments) {
return stepAdjustments(List.of(stepAdjustments));
}
@CustomType.Setter
public Builder target(@Nullable String target) {
this.target = target;
return this;
}
@CustomType.Setter
public Builder threshold(@Nullable Double threshold) {
this.threshold = threshold;
return this;
}
@CustomType.Setter
public Builder unit(@Nullable String unit) {
this.unit = unit;
return this;
}
public ElastigroupScalingUpPolicy build() {
final var _resultValue = new ElastigroupScalingUpPolicy();
_resultValue.actionType = actionType;
_resultValue.adjustment = adjustment;
_resultValue.cooldown = cooldown;
_resultValue.dimensions = dimensions;
_resultValue.evaluationPeriods = evaluationPeriods;
_resultValue.isEnabled = isEnabled;
_resultValue.maxTargetCapacity = maxTargetCapacity;
_resultValue.maximum = maximum;
_resultValue.metricName = metricName;
_resultValue.minTargetCapacity = minTargetCapacity;
_resultValue.minimum = minimum;
_resultValue.namespace = namespace;
_resultValue.operator = operator;
_resultValue.period = period;
_resultValue.policyName = policyName;
_resultValue.source = source;
_resultValue.statistic = statistic;
_resultValue.stepAdjustments = stepAdjustments;
_resultValue.target = target;
_resultValue.threshold = threshold;
_resultValue.unit = unit;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy