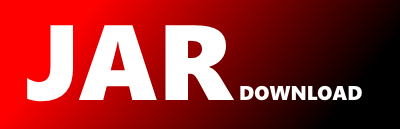
com.pulumi.spotinst.ecs.inputs.OceanState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.ecs.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.spotinst.ecs.inputs.OceanAutoscalerArgs;
import com.pulumi.spotinst.ecs.inputs.OceanBlockDeviceMappingArgs;
import com.pulumi.spotinst.ecs.inputs.OceanClusterOrientationArgs;
import com.pulumi.spotinst.ecs.inputs.OceanFiltersArgs;
import com.pulumi.spotinst.ecs.inputs.OceanInstanceMetadataOptionsArgs;
import com.pulumi.spotinst.ecs.inputs.OceanLoggingArgs;
import com.pulumi.spotinst.ecs.inputs.OceanOptimizeImagesArgs;
import com.pulumi.spotinst.ecs.inputs.OceanScheduledTaskArgs;
import com.pulumi.spotinst.ecs.inputs.OceanTagArgs;
import com.pulumi.spotinst.ecs.inputs.OceanUpdatePolicyArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OceanState extends com.pulumi.resources.ResourceArgs {
public static final OceanState Empty = new OceanState();
/**
* Configure public IP address allocation.
*
*/
@Import(name="associatePublicIpAddress")
private @Nullable Output associatePublicIpAddress;
/**
* @return Configure public IP address allocation.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy