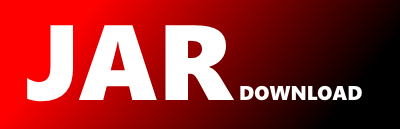
com.pulumi.spotinst.gcp.outputs.ElastigroupScalingUpPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.gcp.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.spotinst.gcp.outputs.ElastigroupScalingUpPolicyDimension;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ElastigroupScalingUpPolicy {
/**
* @return Type of scaling action to take when the scaling policy is triggered. Valid values: "adjustment", "setMinTarget", "updateCapacity", "percentageAdjustment"
*
*/
private @Nullable String actionType;
/**
* @return Value to which the action type will be adjusted. Required if using "numeric" or "percentageAdjustment" action types.
*
*/
private @Nullable Integer adjustment;
/**
* @return Time (seconds) to wait after a scaling action before resuming monitoring.
*
*/
private @Nullable Integer cooldown;
/**
* @return A list of dimensions describing qualities of the metric.
*
*/
private @Nullable List dimensions;
/**
* @return Number of consecutive periods in which the threshold must be met in order to trigger a scaling action.
*
*/
private @Nullable Integer evaluationPeriods;
/**
* @return Metric to monitor. Valid values: "Percentage CPU", "Network In", "Network Out", "Disk Read Bytes", "Disk Write Bytes", "Disk Write Operations/Sec", "Disk Read Operations/Sec".
*
*/
private String metricName;
private String namespace;
/**
* @return The operator used to evaluate the threshold against the current metric value. Valid values: "gt" (greater than), "get" (greater-than or equal), "lt" (less than), "lte" (less than or equal).
*
*/
private @Nullable String operator;
/**
* @return Amount of time (seconds) for which the threshold must be met in order to trigger the scaling action.
*
*/
private @Nullable Integer period;
/**
* @return Name of scaling policy.
*
*/
private String policyName;
/**
* @return Specifies a valid partial or full URL to an existing Persistent Disk resource. This field is only applicable for persistent disks.
*
*/
private @Nullable String source;
/**
* @return Statistic by which to evaluate the selected metric. Valid values: "AVERAGE", "SAMPLE_COUNT", "SUM", "MINIMUM", "MAXIMUM", "PERCENTILE", "COUNT".
*
*/
private @Nullable String statistic;
/**
* @return The value at which the scaling action is triggered.
*
*/
private Double threshold;
private String unit;
private ElastigroupScalingUpPolicy() {}
/**
* @return Type of scaling action to take when the scaling policy is triggered. Valid values: "adjustment", "setMinTarget", "updateCapacity", "percentageAdjustment"
*
*/
public Optional actionType() {
return Optional.ofNullable(this.actionType);
}
/**
* @return Value to which the action type will be adjusted. Required if using "numeric" or "percentageAdjustment" action types.
*
*/
public Optional adjustment() {
return Optional.ofNullable(this.adjustment);
}
/**
* @return Time (seconds) to wait after a scaling action before resuming monitoring.
*
*/
public Optional cooldown() {
return Optional.ofNullable(this.cooldown);
}
/**
* @return A list of dimensions describing qualities of the metric.
*
*/
public List dimensions() {
return this.dimensions == null ? List.of() : this.dimensions;
}
/**
* @return Number of consecutive periods in which the threshold must be met in order to trigger a scaling action.
*
*/
public Optional evaluationPeriods() {
return Optional.ofNullable(this.evaluationPeriods);
}
/**
* @return Metric to monitor. Valid values: "Percentage CPU", "Network In", "Network Out", "Disk Read Bytes", "Disk Write Bytes", "Disk Write Operations/Sec", "Disk Read Operations/Sec".
*
*/
public String metricName() {
return this.metricName;
}
public String namespace() {
return this.namespace;
}
/**
* @return The operator used to evaluate the threshold against the current metric value. Valid values: "gt" (greater than), "get" (greater-than or equal), "lt" (less than), "lte" (less than or equal).
*
*/
public Optional operator() {
return Optional.ofNullable(this.operator);
}
/**
* @return Amount of time (seconds) for which the threshold must be met in order to trigger the scaling action.
*
*/
public Optional period() {
return Optional.ofNullable(this.period);
}
/**
* @return Name of scaling policy.
*
*/
public String policyName() {
return this.policyName;
}
/**
* @return Specifies a valid partial or full URL to an existing Persistent Disk resource. This field is only applicable for persistent disks.
*
*/
public Optional source() {
return Optional.ofNullable(this.source);
}
/**
* @return Statistic by which to evaluate the selected metric. Valid values: "AVERAGE", "SAMPLE_COUNT", "SUM", "MINIMUM", "MAXIMUM", "PERCENTILE", "COUNT".
*
*/
public Optional statistic() {
return Optional.ofNullable(this.statistic);
}
/**
* @return The value at which the scaling action is triggered.
*
*/
public Double threshold() {
return this.threshold;
}
public String unit() {
return this.unit;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ElastigroupScalingUpPolicy defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String actionType;
private @Nullable Integer adjustment;
private @Nullable Integer cooldown;
private @Nullable List dimensions;
private @Nullable Integer evaluationPeriods;
private String metricName;
private String namespace;
private @Nullable String operator;
private @Nullable Integer period;
private String policyName;
private @Nullable String source;
private @Nullable String statistic;
private Double threshold;
private String unit;
public Builder() {}
public Builder(ElastigroupScalingUpPolicy defaults) {
Objects.requireNonNull(defaults);
this.actionType = defaults.actionType;
this.adjustment = defaults.adjustment;
this.cooldown = defaults.cooldown;
this.dimensions = defaults.dimensions;
this.evaluationPeriods = defaults.evaluationPeriods;
this.metricName = defaults.metricName;
this.namespace = defaults.namespace;
this.operator = defaults.operator;
this.period = defaults.period;
this.policyName = defaults.policyName;
this.source = defaults.source;
this.statistic = defaults.statistic;
this.threshold = defaults.threshold;
this.unit = defaults.unit;
}
@CustomType.Setter
public Builder actionType(@Nullable String actionType) {
this.actionType = actionType;
return this;
}
@CustomType.Setter
public Builder adjustment(@Nullable Integer adjustment) {
this.adjustment = adjustment;
return this;
}
@CustomType.Setter
public Builder cooldown(@Nullable Integer cooldown) {
this.cooldown = cooldown;
return this;
}
@CustomType.Setter
public Builder dimensions(@Nullable List dimensions) {
this.dimensions = dimensions;
return this;
}
public Builder dimensions(ElastigroupScalingUpPolicyDimension... dimensions) {
return dimensions(List.of(dimensions));
}
@CustomType.Setter
public Builder evaluationPeriods(@Nullable Integer evaluationPeriods) {
this.evaluationPeriods = evaluationPeriods;
return this;
}
@CustomType.Setter
public Builder metricName(String metricName) {
if (metricName == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "metricName");
}
this.metricName = metricName;
return this;
}
@CustomType.Setter
public Builder namespace(String namespace) {
if (namespace == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "namespace");
}
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder operator(@Nullable String operator) {
this.operator = operator;
return this;
}
@CustomType.Setter
public Builder period(@Nullable Integer period) {
this.period = period;
return this;
}
@CustomType.Setter
public Builder policyName(String policyName) {
if (policyName == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "policyName");
}
this.policyName = policyName;
return this;
}
@CustomType.Setter
public Builder source(@Nullable String source) {
this.source = source;
return this;
}
@CustomType.Setter
public Builder statistic(@Nullable String statistic) {
this.statistic = statistic;
return this;
}
@CustomType.Setter
public Builder threshold(Double threshold) {
if (threshold == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "threshold");
}
this.threshold = threshold;
return this;
}
@CustomType.Setter
public Builder unit(String unit) {
if (unit == null) {
throw new MissingRequiredPropertyException("ElastigroupScalingUpPolicy", "unit");
}
this.unit = unit;
return this;
}
public ElastigroupScalingUpPolicy build() {
final var _resultValue = new ElastigroupScalingUpPolicy();
_resultValue.actionType = actionType;
_resultValue.adjustment = adjustment;
_resultValue.cooldown = cooldown;
_resultValue.dimensions = dimensions;
_resultValue.evaluationPeriods = evaluationPeriods;
_resultValue.metricName = metricName;
_resultValue.namespace = namespace;
_resultValue.operator = operator;
_resultValue.period = period;
_resultValue.policyName = policyName;
_resultValue.source = source;
_resultValue.statistic = statistic;
_resultValue.threshold = threshold;
_resultValue.unit = unit;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy