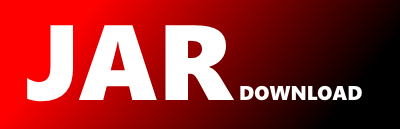
com.pulumi.spotinst.gke.OceanImportArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.gke;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.spotinst.gke.inputs.OceanImportAutoscalerArgs;
import com.pulumi.spotinst.gke.inputs.OceanImportBackendServiceArgs;
import com.pulumi.spotinst.gke.inputs.OceanImportScheduledTaskArgs;
import com.pulumi.spotinst.gke.inputs.OceanImportShieldedInstanceConfigArgs;
import com.pulumi.spotinst.gke.inputs.OceanImportStrategyArgs;
import com.pulumi.spotinst.gke.inputs.OceanImportUpdatePolicyArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OceanImportArgs extends com.pulumi.resources.ResourceArgs {
public static final OceanImportArgs Empty = new OceanImportArgs();
/**
* The Ocean Kubernetes Autoscaler object.
*
*/
@Import(name="autoscaler")
private @Nullable Output autoscaler;
/**
* @return The Ocean Kubernetes Autoscaler object.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy