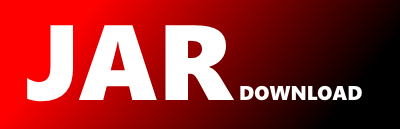
com.pulumi.spotinst.gke.outputs.OceanImportAutoscaler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.gke.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.spotinst.gke.outputs.OceanImportAutoscalerDown;
import com.pulumi.spotinst.gke.outputs.OceanImportAutoscalerHeadroom;
import com.pulumi.spotinst.gke.outputs.OceanImportAutoscalerResourceLimits;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class OceanImportAutoscaler {
/**
* @return Optionally set the auto headroom percentage, set a number between 0-200 to control the headroom % from the cluster. Relevant when isAutoConfig=true.
*
*/
private @Nullable Integer autoHeadroomPercentage;
/**
* @return Cooldown period between scaling actions.
*
*/
private @Nullable Integer cooldown;
/**
* @return Auto Scaling scale down operations.
*
*/
private @Nullable OceanImportAutoscalerDown down;
/**
* @return enables automatic and manual headroom to work in parallel. When set to false, automatic headroom overrides all other headroom definitions manually configured, whether they are at cluster or VNG level.
*
*/
private @Nullable Boolean enableAutomaticAndManualHeadroom;
/**
* @return Spare resource capacity management enabling fast assignment of Pods without waiting for new resources to launch.
*
*/
private @Nullable OceanImportAutoscalerHeadroom headroom;
/**
* @return Automatically configure and optimize headroom resources.
*
*/
private @Nullable Boolean isAutoConfig;
/**
* @return Enable the Ocean Kubernetes Autoscaler.
*
*/
private @Nullable Boolean isEnabled;
/**
* @return Optionally set upper and lower bounds on the resource usage of the cluster.
*
*/
private @Nullable OceanImportAutoscalerResourceLimits resourceLimits;
private OceanImportAutoscaler() {}
/**
* @return Optionally set the auto headroom percentage, set a number between 0-200 to control the headroom % from the cluster. Relevant when isAutoConfig=true.
*
*/
public Optional autoHeadroomPercentage() {
return Optional.ofNullable(this.autoHeadroomPercentage);
}
/**
* @return Cooldown period between scaling actions.
*
*/
public Optional cooldown() {
return Optional.ofNullable(this.cooldown);
}
/**
* @return Auto Scaling scale down operations.
*
*/
public Optional down() {
return Optional.ofNullable(this.down);
}
/**
* @return enables automatic and manual headroom to work in parallel. When set to false, automatic headroom overrides all other headroom definitions manually configured, whether they are at cluster or VNG level.
*
*/
public Optional enableAutomaticAndManualHeadroom() {
return Optional.ofNullable(this.enableAutomaticAndManualHeadroom);
}
/**
* @return Spare resource capacity management enabling fast assignment of Pods without waiting for new resources to launch.
*
*/
public Optional headroom() {
return Optional.ofNullable(this.headroom);
}
/**
* @return Automatically configure and optimize headroom resources.
*
*/
public Optional isAutoConfig() {
return Optional.ofNullable(this.isAutoConfig);
}
/**
* @return Enable the Ocean Kubernetes Autoscaler.
*
*/
public Optional isEnabled() {
return Optional.ofNullable(this.isEnabled);
}
/**
* @return Optionally set upper and lower bounds on the resource usage of the cluster.
*
*/
public Optional resourceLimits() {
return Optional.ofNullable(this.resourceLimits);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(OceanImportAutoscaler defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer autoHeadroomPercentage;
private @Nullable Integer cooldown;
private @Nullable OceanImportAutoscalerDown down;
private @Nullable Boolean enableAutomaticAndManualHeadroom;
private @Nullable OceanImportAutoscalerHeadroom headroom;
private @Nullable Boolean isAutoConfig;
private @Nullable Boolean isEnabled;
private @Nullable OceanImportAutoscalerResourceLimits resourceLimits;
public Builder() {}
public Builder(OceanImportAutoscaler defaults) {
Objects.requireNonNull(defaults);
this.autoHeadroomPercentage = defaults.autoHeadroomPercentage;
this.cooldown = defaults.cooldown;
this.down = defaults.down;
this.enableAutomaticAndManualHeadroom = defaults.enableAutomaticAndManualHeadroom;
this.headroom = defaults.headroom;
this.isAutoConfig = defaults.isAutoConfig;
this.isEnabled = defaults.isEnabled;
this.resourceLimits = defaults.resourceLimits;
}
@CustomType.Setter
public Builder autoHeadroomPercentage(@Nullable Integer autoHeadroomPercentage) {
this.autoHeadroomPercentage = autoHeadroomPercentage;
return this;
}
@CustomType.Setter
public Builder cooldown(@Nullable Integer cooldown) {
this.cooldown = cooldown;
return this;
}
@CustomType.Setter
public Builder down(@Nullable OceanImportAutoscalerDown down) {
this.down = down;
return this;
}
@CustomType.Setter
public Builder enableAutomaticAndManualHeadroom(@Nullable Boolean enableAutomaticAndManualHeadroom) {
this.enableAutomaticAndManualHeadroom = enableAutomaticAndManualHeadroom;
return this;
}
@CustomType.Setter
public Builder headroom(@Nullable OceanImportAutoscalerHeadroom headroom) {
this.headroom = headroom;
return this;
}
@CustomType.Setter
public Builder isAutoConfig(@Nullable Boolean isAutoConfig) {
this.isAutoConfig = isAutoConfig;
return this;
}
@CustomType.Setter
public Builder isEnabled(@Nullable Boolean isEnabled) {
this.isEnabled = isEnabled;
return this;
}
@CustomType.Setter
public Builder resourceLimits(@Nullable OceanImportAutoscalerResourceLimits resourceLimits) {
this.resourceLimits = resourceLimits;
return this;
}
public OceanImportAutoscaler build() {
final var _resultValue = new OceanImportAutoscaler();
_resultValue.autoHeadroomPercentage = autoHeadroomPercentage;
_resultValue.cooldown = cooldown;
_resultValue.down = down;
_resultValue.enableAutomaticAndManualHeadroom = enableAutomaticAndManualHeadroom;
_resultValue.headroom = headroom;
_resultValue.isAutoConfig = isAutoConfig;
_resultValue.isEnabled = isEnabled;
_resultValue.resourceLimits = resourceLimits;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy