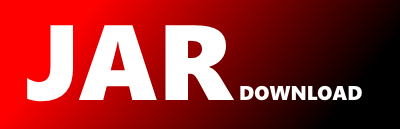
com.pulumi.spotinst.oceancd.inputs.RolloutSpecState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.oceancd.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.spotinst.oceancd.inputs.RolloutSpecFailurePolicyArgs;
import com.pulumi.spotinst.oceancd.inputs.RolloutSpecSpotDeploymentArgs;
import com.pulumi.spotinst.oceancd.inputs.RolloutSpecStrategyArgs;
import com.pulumi.spotinst.oceancd.inputs.RolloutSpecTrafficArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RolloutSpecState extends com.pulumi.resources.ResourceArgs {
public static final RolloutSpecState Empty = new RolloutSpecState();
/**
* Holds information on how to react when failure happens.
*
*/
@Import(name="failurePolicy")
private @Nullable Output failurePolicy;
/**
* @return Holds information on how to react when failure happens.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy