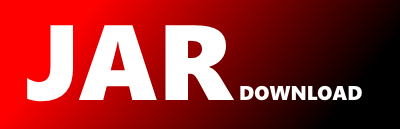
com.pulumi.spotinst.organization.inputs.UserState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spotinst Show documentation
Show all versions of spotinst Show documentation
A Pulumi package for creating and managing spotinst cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.spotinst.organization.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.spotinst.organization.inputs.UserPolicyArgs;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UserState extends com.pulumi.resources.ResourceArgs {
public static final UserState Empty = new UserState();
/**
* Email.
*
*/
@Import(name="email")
private @Nullable Output email;
/**
* @return Email.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy