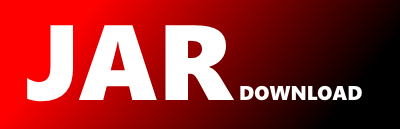
com.pulumi.sumologic.CloudwatchSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sumologic Show documentation
Show all versions of sumologic Show documentation
A Pulumi package for creating and managing sumologic cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.sumologic;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.sumologic.CloudwatchSourceArgs;
import com.pulumi.sumologic.Utilities;
import com.pulumi.sumologic.inputs.CloudwatchSourceState;
import com.pulumi.sumologic.outputs.CloudwatchSourceAuthentication;
import com.pulumi.sumologic.outputs.CloudwatchSourceDefaultDateFormat;
import com.pulumi.sumologic.outputs.CloudwatchSourceFilter;
import com.pulumi.sumologic.outputs.CloudwatchSourcePath;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a [Sumologic CloudWatch source](https://help.sumologic.com/03Send-Data/Sources/02Sources-for-Hosted-Collectors/Amazon-Web-Services/Amazon-CloudWatch-Source-for-Metrics).
*
* __IMPORTANT:__ The AWS credentials are stored in plain-text in the state. This is a potential security issue.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* CloudWatch sources can be imported using the collector and source IDs (`collector/source`), e.g.:
*
* hcl
*
* ```sh
* $ pulumi import sumologic:index/cloudwatchSource:CloudwatchSource test 123/456
* ```
*
* CloudWatch sources can be imported using the collector name and source name (`collectorName/sourceName`), e.g.:
*
* hcl
*
* ```sh
* $ pulumi import sumologic:index/cloudwatchSource:CloudwatchSource test my-test-collector/my-test-source
* ```
*
* [1]: https://help.sumologic.com/Send_Data/Sources/03Use_JSON_to_Configure_Sources/JSON_Parameters_for_Hosted_Sources
*
* [2]: https://help.sumologic.com/03Send-Data/Sources/02Sources-for-Hosted-Collectors/Amazon-Web-Services/Amazon-CloudWatch-Source-for-Metrics
*
*/
@ResourceType(type="sumologic:index/cloudwatchSource:CloudwatchSource")
public class CloudwatchSource extends com.pulumi.resources.CustomResource {
/**
* Authentication details for connecting to the S3 bucket.
*
*/
@Export(name="authentication", refs={CloudwatchSourceAuthentication.class}, tree="[0]")
private Output authentication;
/**
* @return Authentication details for connecting to the S3 bucket.
*
*/
public Output authentication() {
return this.authentication;
}
@Export(name="automaticDateParsing", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> automaticDateParsing;
public Output> automaticDateParsing() {
return Codegen.optional(this.automaticDateParsing);
}
@Export(name="category", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> category;
public Output> category() {
return Codegen.optional(this.category);
}
@Export(name="collectorId", refs={Integer.class}, tree="[0]")
private Output collectorId;
public Output collectorId() {
return this.collectorId;
}
/**
* The content-type of the collected data. Details can be found in the [Sumologic documentation for hosted sources](https://help.sumologic.com/Send_Data/Sources/03Use_JSON_to_Configure_Sources/JSON_Parameters_for_Hosted_Sources).
*
*/
@Export(name="contentType", refs={String.class}, tree="[0]")
private Output contentType;
/**
* @return The content-type of the collected data. Details can be found in the [Sumologic documentation for hosted sources](https://help.sumologic.com/Send_Data/Sources/03Use_JSON_to_Configure_Sources/JSON_Parameters_for_Hosted_Sources).
*
*/
public Output contentType() {
return this.contentType;
}
@Export(name="cutoffRelativeTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> cutoffRelativeTime;
public Output> cutoffRelativeTime() {
return Codegen.optional(this.cutoffRelativeTime);
}
@Export(name="cutoffTimestamp", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> cutoffTimestamp;
public Output> cutoffTimestamp() {
return Codegen.optional(this.cutoffTimestamp);
}
@Export(name="defaultDateFormats", refs={List.class,CloudwatchSourceDefaultDateFormat.class}, tree="[0,1]")
private Output* @Nullable */ List> defaultDateFormats;
public Output>> defaultDateFormats() {
return Codegen.optional(this.defaultDateFormats);
}
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
public Output> description() {
return Codegen.optional(this.description);
}
@Export(name="fields", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> fields;
public Output>> fields() {
return Codegen.optional(this.fields);
}
@Export(name="filters", refs={List.class,CloudwatchSourceFilter.class}, tree="[0,1]")
private Output* @Nullable */ List> filters;
public Output>> filters() {
return Codegen.optional(this.filters);
}
@Export(name="forceTimezone", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceTimezone;
public Output> forceTimezone() {
return Codegen.optional(this.forceTimezone);
}
@Export(name="hashAlgorithm", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> hashAlgorithm;
public Output> hashAlgorithm() {
return Codegen.optional(this.hashAlgorithm);
}
@Export(name="hostName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> hostName;
public Output> hostName() {
return Codegen.optional(this.hostName);
}
@Export(name="manualPrefixRegexp", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> manualPrefixRegexp;
public Output> manualPrefixRegexp() {
return Codegen.optional(this.manualPrefixRegexp);
}
@Export(name="multilineProcessingEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> multilineProcessingEnabled;
public Output> multilineProcessingEnabled() {
return Codegen.optional(this.multilineProcessingEnabled);
}
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
public Output name() {
return this.name;
}
/**
* The location to scan for new data.
*
*/
@Export(name="path", refs={CloudwatchSourcePath.class}, tree="[0]")
private Output path;
/**
* @return The location to scan for new data.
*
*/
public Output path() {
return this.path;
}
/**
* When set to true, the scanner is paused. To disable, set to false.
*
*/
@Export(name="paused", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> paused;
/**
* @return When set to true, the scanner is paused. To disable, set to false.
*
*/
public Output> paused() {
return Codegen.optional(this.paused);
}
/**
* Time interval in milliseconds of scans for new data. The default is 300000 and the minimum value is 1000 milliseconds.
*
*/
@Export(name="scanInterval", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> scanInterval;
/**
* @return Time interval in milliseconds of scans for new data. The default is 300000 and the minimum value is 1000 milliseconds.
*
*/
public Output> scanInterval() {
return Codegen.optional(this.scanInterval);
}
@Export(name="timezone", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> timezone;
public Output> timezone() {
return Codegen.optional(this.timezone);
}
/**
* The HTTP endpoint to use with [SNS to notify Sumo Logic of new files](<https://help.sumologic.com/03Send-Data/Sources/02Sources-for-Hosted-Collectors/Amazon-Web-Services/AWS-S3-Source#Set_up_SNS_in_AWS_(Optional)>).
*
*/
@Export(name="url", refs={String.class}, tree="[0]")
private Output url;
/**
* @return The HTTP endpoint to use with [SNS to notify Sumo Logic of new files](<https://help.sumologic.com/03Send-Data/Sources/02Sources-for-Hosted-Collectors/Amazon-Web-Services/AWS-S3-Source#Set_up_SNS_in_AWS_(Optional)>).
*
*/
public Output url() {
return this.url;
}
@Export(name="useAutolineMatching", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> useAutolineMatching;
public Output> useAutolineMatching() {
return Codegen.optional(this.useAutolineMatching);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public CloudwatchSource(java.lang.String name) {
this(name, CloudwatchSourceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public CloudwatchSource(java.lang.String name, CloudwatchSourceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public CloudwatchSource(java.lang.String name, CloudwatchSourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("sumologic:index/cloudwatchSource:CloudwatchSource", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private CloudwatchSource(java.lang.String name, Output id, @Nullable CloudwatchSourceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("sumologic:index/cloudwatchSource:CloudwatchSource", name, state, makeResourceOptions(options, id), false);
}
private static CloudwatchSourceArgs makeArgs(CloudwatchSourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? CloudwatchSourceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static CloudwatchSource get(java.lang.String name, Output id, @Nullable CloudwatchSourceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new CloudwatchSource(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy