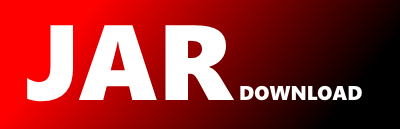
com.pulumi.sumologic.HttpSourceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.sumologic;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.sumologic.inputs.HttpSourceDefaultDateFormatArgs;
import com.pulumi.sumologic.inputs.HttpSourceFilterArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class HttpSourceArgs extends com.pulumi.resources.ResourceArgs {
public static final HttpSourceArgs Empty = new HttpSourceArgs();
@Import(name="automaticDateParsing")
private @Nullable Output automaticDateParsing;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy