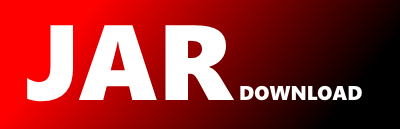
com.pulumi.sumologic.inputs.CloudwatchSourcePathArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sumologic Show documentation
Show all versions of sumologic Show documentation
A Pulumi package for creating and managing sumologic cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.sumologic.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.sumologic.inputs.CloudwatchSourcePathCustomServiceArgs;
import com.pulumi.sumologic.inputs.CloudwatchSourcePathSnsTopicOrSubscriptionArnArgs;
import com.pulumi.sumologic.inputs.CloudwatchSourcePathTagFilterArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CloudwatchSourcePathArgs extends com.pulumi.resources.ResourceArgs {
public static final CloudwatchSourcePathArgs Empty = new CloudwatchSourcePathArgs();
@Import(name="bucketName")
private @Nullable Output bucketName;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy