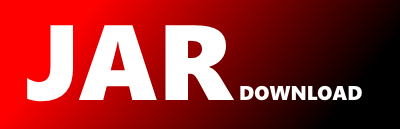
com.pulumi.sumologic.inputs.MonitorFolderState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sumologic Show documentation
Show all versions of sumologic Show documentation
A Pulumi package for creating and managing sumologic cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.sumologic.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.sumologic.inputs.MonitorFolderObjPermissionArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MonitorFolderState extends com.pulumi.resources.ResourceArgs {
public static final MonitorFolderState Empty = new MonitorFolderState();
@Import(name="contentType")
private @Nullable Output contentType;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy