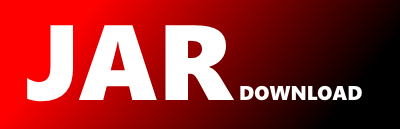
com.pulumi.sumologic.outputs.CloudfrontSourcePath Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.sumologic.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.sumologic.outputs.CloudfrontSourcePathCustomService;
import com.pulumi.sumologic.outputs.CloudfrontSourcePathSnsTopicOrSubscriptionArn;
import com.pulumi.sumologic.outputs.CloudfrontSourcePathTagFilter;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CloudfrontSourcePath {
/**
* @return The name of the bucket. This is needed if using type `S3BucketPathExpression`.
*
*/
private @Nullable String bucketName;
private @Nullable String consumerGroup;
private @Nullable List customServices;
private @Nullable String eventHubName;
private @Nullable List limitToNamespaces;
private @Nullable List limitToRegions;
private @Nullable List limitToServices;
private @Nullable String namespace;
/**
* @return The path to the data. This is needed if using type `S3BucketPathExpression`.
*
*/
private @Nullable String pathExpression;
/**
* @return Your AWS Bucket region.
*
*/
private @Nullable String region;
/**
* @return This is a computed field for SNS topic/subscription ARN.
*
*/
private @Nullable List snsTopicOrSubscriptionArns;
private @Nullable List tagFilters;
/**
* @return type of polling source. This has to be `S3BucketPathExpression` for `CloudFront` source.
*
*/
private String type;
private @Nullable Boolean useVersionedApi;
private CloudfrontSourcePath() {}
/**
* @return The name of the bucket. This is needed if using type `S3BucketPathExpression`.
*
*/
public Optional bucketName() {
return Optional.ofNullable(this.bucketName);
}
public Optional consumerGroup() {
return Optional.ofNullable(this.consumerGroup);
}
public List customServices() {
return this.customServices == null ? List.of() : this.customServices;
}
public Optional eventHubName() {
return Optional.ofNullable(this.eventHubName);
}
public List limitToNamespaces() {
return this.limitToNamespaces == null ? List.of() : this.limitToNamespaces;
}
public List limitToRegions() {
return this.limitToRegions == null ? List.of() : this.limitToRegions;
}
public List limitToServices() {
return this.limitToServices == null ? List.of() : this.limitToServices;
}
public Optional namespace() {
return Optional.ofNullable(this.namespace);
}
/**
* @return The path to the data. This is needed if using type `S3BucketPathExpression`.
*
*/
public Optional pathExpression() {
return Optional.ofNullable(this.pathExpression);
}
/**
* @return Your AWS Bucket region.
*
*/
public Optional region() {
return Optional.ofNullable(this.region);
}
/**
* @return This is a computed field for SNS topic/subscription ARN.
*
*/
public List snsTopicOrSubscriptionArns() {
return this.snsTopicOrSubscriptionArns == null ? List.of() : this.snsTopicOrSubscriptionArns;
}
public List tagFilters() {
return this.tagFilters == null ? List.of() : this.tagFilters;
}
/**
* @return type of polling source. This has to be `S3BucketPathExpression` for `CloudFront` source.
*
*/
public String type() {
return this.type;
}
public Optional useVersionedApi() {
return Optional.ofNullable(this.useVersionedApi);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CloudfrontSourcePath defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String bucketName;
private @Nullable String consumerGroup;
private @Nullable List customServices;
private @Nullable String eventHubName;
private @Nullable List limitToNamespaces;
private @Nullable List limitToRegions;
private @Nullable List limitToServices;
private @Nullable String namespace;
private @Nullable String pathExpression;
private @Nullable String region;
private @Nullable List snsTopicOrSubscriptionArns;
private @Nullable List tagFilters;
private String type;
private @Nullable Boolean useVersionedApi;
public Builder() {}
public Builder(CloudfrontSourcePath defaults) {
Objects.requireNonNull(defaults);
this.bucketName = defaults.bucketName;
this.consumerGroup = defaults.consumerGroup;
this.customServices = defaults.customServices;
this.eventHubName = defaults.eventHubName;
this.limitToNamespaces = defaults.limitToNamespaces;
this.limitToRegions = defaults.limitToRegions;
this.limitToServices = defaults.limitToServices;
this.namespace = defaults.namespace;
this.pathExpression = defaults.pathExpression;
this.region = defaults.region;
this.snsTopicOrSubscriptionArns = defaults.snsTopicOrSubscriptionArns;
this.tagFilters = defaults.tagFilters;
this.type = defaults.type;
this.useVersionedApi = defaults.useVersionedApi;
}
@CustomType.Setter
public Builder bucketName(@Nullable String bucketName) {
this.bucketName = bucketName;
return this;
}
@CustomType.Setter
public Builder consumerGroup(@Nullable String consumerGroup) {
this.consumerGroup = consumerGroup;
return this;
}
@CustomType.Setter
public Builder customServices(@Nullable List customServices) {
this.customServices = customServices;
return this;
}
public Builder customServices(CloudfrontSourcePathCustomService... customServices) {
return customServices(List.of(customServices));
}
@CustomType.Setter
public Builder eventHubName(@Nullable String eventHubName) {
this.eventHubName = eventHubName;
return this;
}
@CustomType.Setter
public Builder limitToNamespaces(@Nullable List limitToNamespaces) {
this.limitToNamespaces = limitToNamespaces;
return this;
}
public Builder limitToNamespaces(String... limitToNamespaces) {
return limitToNamespaces(List.of(limitToNamespaces));
}
@CustomType.Setter
public Builder limitToRegions(@Nullable List limitToRegions) {
this.limitToRegions = limitToRegions;
return this;
}
public Builder limitToRegions(String... limitToRegions) {
return limitToRegions(List.of(limitToRegions));
}
@CustomType.Setter
public Builder limitToServices(@Nullable List limitToServices) {
this.limitToServices = limitToServices;
return this;
}
public Builder limitToServices(String... limitToServices) {
return limitToServices(List.of(limitToServices));
}
@CustomType.Setter
public Builder namespace(@Nullable String namespace) {
this.namespace = namespace;
return this;
}
@CustomType.Setter
public Builder pathExpression(@Nullable String pathExpression) {
this.pathExpression = pathExpression;
return this;
}
@CustomType.Setter
public Builder region(@Nullable String region) {
this.region = region;
return this;
}
@CustomType.Setter
public Builder snsTopicOrSubscriptionArns(@Nullable List snsTopicOrSubscriptionArns) {
this.snsTopicOrSubscriptionArns = snsTopicOrSubscriptionArns;
return this;
}
public Builder snsTopicOrSubscriptionArns(CloudfrontSourcePathSnsTopicOrSubscriptionArn... snsTopicOrSubscriptionArns) {
return snsTopicOrSubscriptionArns(List.of(snsTopicOrSubscriptionArns));
}
@CustomType.Setter
public Builder tagFilters(@Nullable List tagFilters) {
this.tagFilters = tagFilters;
return this;
}
public Builder tagFilters(CloudfrontSourcePathTagFilter... tagFilters) {
return tagFilters(List.of(tagFilters));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("CloudfrontSourcePath", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder useVersionedApi(@Nullable Boolean useVersionedApi) {
this.useVersionedApi = useVersionedApi;
return this;
}
public CloudfrontSourcePath build() {
final var _resultValue = new CloudfrontSourcePath();
_resultValue.bucketName = bucketName;
_resultValue.consumerGroup = consumerGroup;
_resultValue.customServices = customServices;
_resultValue.eventHubName = eventHubName;
_resultValue.limitToNamespaces = limitToNamespaces;
_resultValue.limitToRegions = limitToRegions;
_resultValue.limitToServices = limitToServices;
_resultValue.namespace = namespace;
_resultValue.pathExpression = pathExpression;
_resultValue.region = region;
_resultValue.snsTopicOrSubscriptionArns = snsTopicOrSubscriptionArns;
_resultValue.tagFilters = tagFilters;
_resultValue.type = type;
_resultValue.useVersionedApi = useVersionedApi;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy