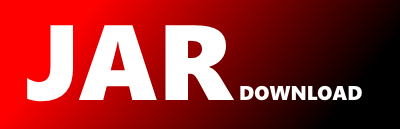
com.pulumi.vault.MfaDuoArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MfaDuoArgs extends com.pulumi.resources.ResourceArgs {
public static final MfaDuoArgs Empty = new MfaDuoArgs();
/**
* `(string: <required>)` - API hostname for Duo.
*
*/
@Import(name="apiHostname", required=true)
private Output apiHostname;
/**
* @return `(string: <required>)` - API hostname for Duo.
*
*/
public Output apiHostname() {
return this.apiHostname;
}
/**
* `(string: <required>)` - Integration key for Duo.
*
*/
@Import(name="integrationKey", required=true)
private Output integrationKey;
/**
* @return `(string: <required>)` - Integration key for Duo.
*
*/
public Output integrationKey() {
return this.integrationKey;
}
/**
* `(string: <required>)` - The mount to tie this method to for use in automatic mappings. The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*
*/
@Import(name="mountAccessor", required=true)
private Output mountAccessor;
/**
* @return `(string: <required>)` - The mount to tie this method to for use in automatic mappings. The mapping will use the Name field of Aliases associated with this mount as the username in the mapping.
*
*/
public Output mountAccessor() {
return this.mountAccessor;
}
/**
* `(string: <required>)` – Name of the MFA method.
*
*/
@Import(name="name")
private @Nullable Output name;
/**
* @return `(string: <required>)` – Name of the MFA method.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy