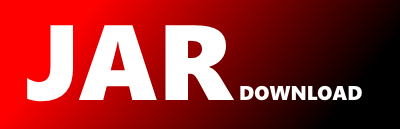
com.pulumi.vault.MfaTotpArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class MfaTotpArgs extends com.pulumi.resources.ResourceArgs {
public static final MfaTotpArgs Empty = new MfaTotpArgs();
/**
* `(string)` - Specifies the hashing algorithm used to generate the TOTP code.
* Options include `SHA1`, `SHA256` and `SHA512`
*
*/
@Import(name="algorithm")
private @Nullable Output algorithm;
/**
* @return `(string)` - Specifies the hashing algorithm used to generate the TOTP code.
* Options include `SHA1`, `SHA256` and `SHA512`
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy