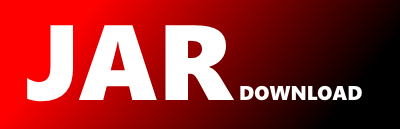
com.pulumi.vault.appRole.inputs.AuthBackendRoleSecretIdState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.appRole.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AuthBackendRoleSecretIdState extends com.pulumi.resources.ResourceArgs {
public static final AuthBackendRoleSecretIdState Empty = new AuthBackendRoleSecretIdState();
/**
* The unique ID for this SecretID that can be safely logged.
*
*/
@Import(name="accessor")
private @Nullable Output accessor;
/**
* @return The unique ID for this SecretID that can be safely logged.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy