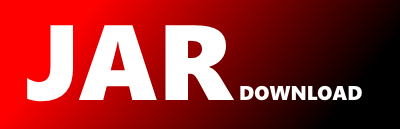
com.pulumi.vault.database.inputs.SecretsMountMysqlArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.database.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SecretsMountMysqlArgs extends com.pulumi.resources.ResourceArgs {
public static final SecretsMountMysqlArgs Empty = new SecretsMountMysqlArgs();
/**
* A list of roles that are allowed to use this
* connection.
*
*/
@Import(name="allowedRoles")
private @Nullable Output> allowedRoles;
/**
* @return A list of roles that are allowed to use this
* connection.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy