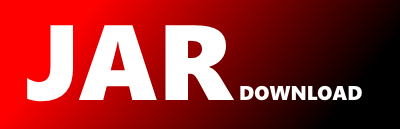
com.pulumi.vault.database.outputs.SecretsMountOracle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.database.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SecretsMountOracle {
/**
* @return A list of roles that are allowed to use this
* connection.
*
*/
private @Nullable List allowedRoles;
/**
* @return Connection string to use to connect to the database.
*
*/
private @Nullable String connectionUrl;
/**
* @return A map of sensitive data to pass to the endpoint. Useful for templated connection strings.
*
* Supported list of database secrets engines that can be configured:
*
*/
private @Nullable Map data;
/**
* @return Set to true to disconnect any open sessions prior to running the revocation statements.
*
*/
private @Nullable Boolean disconnectSessions;
/**
* @return Maximum number of seconds a connection may be reused.
*
*/
private @Nullable Integer maxConnectionLifetime;
/**
* @return Maximum number of idle connections to the database.
*
*/
private @Nullable Integer maxIdleConnections;
/**
* @return Maximum number of open connections to the database.
*
*/
private @Nullable Integer maxOpenConnections;
/**
* @return Name of the database connection.
*
*/
private String name;
/**
* @return The root credential password used in the connection URL
*
*/
private @Nullable String password;
/**
* @return Specifies the name of the plugin to use.
*
*/
private @Nullable String pluginName;
/**
* @return A list of database statements to be executed to rotate the root user's credentials.
*
*/
private @Nullable List rootRotationStatements;
/**
* @return Set to true in order to split statements after semi-colons.
*
*/
private @Nullable Boolean splitStatements;
/**
* @return The root credential username used in the connection URL
*
*/
private @Nullable String username;
/**
* @return Username generation template.
*
*/
private @Nullable String usernameTemplate;
/**
* @return Whether the connection should be verified on
* initial configuration or not.
*
*/
private @Nullable Boolean verifyConnection;
private SecretsMountOracle() {}
/**
* @return A list of roles that are allowed to use this
* connection.
*
*/
public List allowedRoles() {
return this.allowedRoles == null ? List.of() : this.allowedRoles;
}
/**
* @return Connection string to use to connect to the database.
*
*/
public Optional connectionUrl() {
return Optional.ofNullable(this.connectionUrl);
}
/**
* @return A map of sensitive data to pass to the endpoint. Useful for templated connection strings.
*
* Supported list of database secrets engines that can be configured:
*
*/
public Map data() {
return this.data == null ? Map.of() : this.data;
}
/**
* @return Set to true to disconnect any open sessions prior to running the revocation statements.
*
*/
public Optional disconnectSessions() {
return Optional.ofNullable(this.disconnectSessions);
}
/**
* @return Maximum number of seconds a connection may be reused.
*
*/
public Optional maxConnectionLifetime() {
return Optional.ofNullable(this.maxConnectionLifetime);
}
/**
* @return Maximum number of idle connections to the database.
*
*/
public Optional maxIdleConnections() {
return Optional.ofNullable(this.maxIdleConnections);
}
/**
* @return Maximum number of open connections to the database.
*
*/
public Optional maxOpenConnections() {
return Optional.ofNullable(this.maxOpenConnections);
}
/**
* @return Name of the database connection.
*
*/
public String name() {
return this.name;
}
/**
* @return The root credential password used in the connection URL
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return Specifies the name of the plugin to use.
*
*/
public Optional pluginName() {
return Optional.ofNullable(this.pluginName);
}
/**
* @return A list of database statements to be executed to rotate the root user's credentials.
*
*/
public List rootRotationStatements() {
return this.rootRotationStatements == null ? List.of() : this.rootRotationStatements;
}
/**
* @return Set to true in order to split statements after semi-colons.
*
*/
public Optional splitStatements() {
return Optional.ofNullable(this.splitStatements);
}
/**
* @return The root credential username used in the connection URL
*
*/
public Optional username() {
return Optional.ofNullable(this.username);
}
/**
* @return Username generation template.
*
*/
public Optional usernameTemplate() {
return Optional.ofNullable(this.usernameTemplate);
}
/**
* @return Whether the connection should be verified on
* initial configuration or not.
*
*/
public Optional verifyConnection() {
return Optional.ofNullable(this.verifyConnection);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SecretsMountOracle defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List allowedRoles;
private @Nullable String connectionUrl;
private @Nullable Map data;
private @Nullable Boolean disconnectSessions;
private @Nullable Integer maxConnectionLifetime;
private @Nullable Integer maxIdleConnections;
private @Nullable Integer maxOpenConnections;
private String name;
private @Nullable String password;
private @Nullable String pluginName;
private @Nullable List rootRotationStatements;
private @Nullable Boolean splitStatements;
private @Nullable String username;
private @Nullable String usernameTemplate;
private @Nullable Boolean verifyConnection;
public Builder() {}
public Builder(SecretsMountOracle defaults) {
Objects.requireNonNull(defaults);
this.allowedRoles = defaults.allowedRoles;
this.connectionUrl = defaults.connectionUrl;
this.data = defaults.data;
this.disconnectSessions = defaults.disconnectSessions;
this.maxConnectionLifetime = defaults.maxConnectionLifetime;
this.maxIdleConnections = defaults.maxIdleConnections;
this.maxOpenConnections = defaults.maxOpenConnections;
this.name = defaults.name;
this.password = defaults.password;
this.pluginName = defaults.pluginName;
this.rootRotationStatements = defaults.rootRotationStatements;
this.splitStatements = defaults.splitStatements;
this.username = defaults.username;
this.usernameTemplate = defaults.usernameTemplate;
this.verifyConnection = defaults.verifyConnection;
}
@CustomType.Setter
public Builder allowedRoles(@Nullable List allowedRoles) {
this.allowedRoles = allowedRoles;
return this;
}
public Builder allowedRoles(String... allowedRoles) {
return allowedRoles(List.of(allowedRoles));
}
@CustomType.Setter
public Builder connectionUrl(@Nullable String connectionUrl) {
this.connectionUrl = connectionUrl;
return this;
}
@CustomType.Setter
public Builder data(@Nullable Map data) {
this.data = data;
return this;
}
@CustomType.Setter
public Builder disconnectSessions(@Nullable Boolean disconnectSessions) {
this.disconnectSessions = disconnectSessions;
return this;
}
@CustomType.Setter
public Builder maxConnectionLifetime(@Nullable Integer maxConnectionLifetime) {
this.maxConnectionLifetime = maxConnectionLifetime;
return this;
}
@CustomType.Setter
public Builder maxIdleConnections(@Nullable Integer maxIdleConnections) {
this.maxIdleConnections = maxIdleConnections;
return this;
}
@CustomType.Setter
public Builder maxOpenConnections(@Nullable Integer maxOpenConnections) {
this.maxOpenConnections = maxOpenConnections;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("SecretsMountOracle", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder pluginName(@Nullable String pluginName) {
this.pluginName = pluginName;
return this;
}
@CustomType.Setter
public Builder rootRotationStatements(@Nullable List rootRotationStatements) {
this.rootRotationStatements = rootRotationStatements;
return this;
}
public Builder rootRotationStatements(String... rootRotationStatements) {
return rootRotationStatements(List.of(rootRotationStatements));
}
@CustomType.Setter
public Builder splitStatements(@Nullable Boolean splitStatements) {
this.splitStatements = splitStatements;
return this;
}
@CustomType.Setter
public Builder username(@Nullable String username) {
this.username = username;
return this;
}
@CustomType.Setter
public Builder usernameTemplate(@Nullable String usernameTemplate) {
this.usernameTemplate = usernameTemplate;
return this;
}
@CustomType.Setter
public Builder verifyConnection(@Nullable Boolean verifyConnection) {
this.verifyConnection = verifyConnection;
return this;
}
public SecretsMountOracle build() {
final var _resultValue = new SecretsMountOracle();
_resultValue.allowedRoles = allowedRoles;
_resultValue.connectionUrl = connectionUrl;
_resultValue.data = data;
_resultValue.disconnectSessions = disconnectSessions;
_resultValue.maxConnectionLifetime = maxConnectionLifetime;
_resultValue.maxIdleConnections = maxIdleConnections;
_resultValue.maxOpenConnections = maxOpenConnections;
_resultValue.name = name;
_resultValue.password = password;
_resultValue.pluginName = pluginName;
_resultValue.rootRotationStatements = rootRotationStatements;
_resultValue.splitStatements = splitStatements;
_resultValue.username = username;
_resultValue.usernameTemplate = usernameTemplate;
_resultValue.verifyConnection = verifyConnection;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy