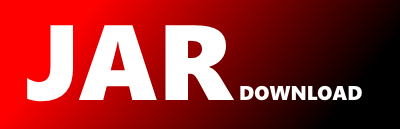
com.pulumi.vault.generic.Endpoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.generic;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.vault.Utilities;
import com.pulumi.vault.generic.EndpointArgs;
import com.pulumi.vault.generic.inputs.EndpointState;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.generic.Endpoint;
* import com.pulumi.vault.generic.EndpointArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var userpass = new AuthBackend("userpass", AuthBackendArgs.builder()
* .type("userpass")
* .build());
*
* var u1 = new Endpoint("u1", EndpointArgs.builder()
* .path("auth/userpass/users/u1")
* .ignoreAbsentFields(true)
* .dataJson("""
* {
* "policies": ["p1"],
* "password": "changeme"
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(userpass)
* .build());
*
* var u1Token = new Endpoint("u1Token", EndpointArgs.builder()
* .path("auth/userpass/login/u1")
* .disableRead(true)
* .disableDelete(true)
* .dataJson("""
* {
* "password": "changeme"
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(u1)
* .build());
*
* var u1Entity = new Endpoint("u1Entity", EndpointArgs.builder()
* .disableRead(true)
* .disableDelete(true)
* .path("identity/lookup/entity")
* .ignoreAbsentFields(true)
* .writeFields("id")
* .dataJson("""
* {
* "alias_name": "u1",
* "alias_mount_accessor": vault_auth_backend.userpass.accessor
* }
* """)
* .build(), CustomResourceOptions.builder()
* .dependsOn(u1Token)
* .build());
*
* ctx.export("u1Id", u1Entity.writeData().applyValue(writeData -> writeData.id()));
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Required Vault Capabilities
*
* Use of this resource requires the `create` or `update` capability
* (depending on whether the resource already exists) on the given path. If
* `disable_delete` is false, the `delete` capability is also required. If
* `disable_read` is false, the `read` capability is required.
*
* ## Import
*
* Import is not supported for this resource.
*
*/
@ResourceType(type="vault:generic/endpoint:Endpoint")
public class Endpoint extends com.pulumi.resources.CustomResource {
/**
* String containing a JSON-encoded object that will be
* written to the given path as the secret data.
*
*/
@Export(name="dataJson", refs={String.class}, tree="[0]")
private Output dataJson;
/**
* @return String containing a JSON-encoded object that will be
* written to the given path as the secret data.
*
*/
public Output dataJson() {
return this.dataJson;
}
/**
* - (Optional) True/false. Set this to true if your
* vault authentication is not able to delete the data or if the endpoint
* does not support the `DELETE` method. Defaults to false.
*
*/
@Export(name="disableDelete", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableDelete;
/**
* @return - (Optional) True/false. Set this to true if your
* vault authentication is not able to delete the data or if the endpoint
* does not support the `DELETE` method. Defaults to false.
*
*/
public Output> disableDelete() {
return Codegen.optional(this.disableDelete);
}
/**
* True/false. Set this to true if your vault
* authentication is not able to read the data or if the endpoint does
* not support the `GET` method. Setting this to `true` will break drift
* detection. You should set this to `true` for endpoints that are
* write-only. Defaults to false.
*
*/
@Export(name="disableRead", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableRead;
/**
* @return True/false. Set this to true if your vault
* authentication is not able to read the data or if the endpoint does
* not support the `GET` method. Setting this to `true` will break drift
* detection. You should set this to `true` for endpoints that are
* write-only. Defaults to false.
*
*/
public Output> disableRead() {
return Codegen.optional(this.disableRead);
}
/**
* - (Optional) True/false. If set to true,
* ignore any fields present when the endpoint is read but that were not
* in `data_json`. Also, if a field that was written is not returned when
* the endpoint is read, treat that field as being up to date. You should
* set this to `true` when writing to endpoint that, when read, returns a
* different set of fields from the ones you wrote, as is common with
* many configuration endpoints. Defaults to false.
*
*/
@Export(name="ignoreAbsentFields", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> ignoreAbsentFields;
/**
* @return - (Optional) True/false. If set to true,
* ignore any fields present when the endpoint is read but that were not
* in `data_json`. Also, if a field that was written is not returned when
* the endpoint is read, treat that field as being up to date. You should
* set this to `true` when writing to endpoint that, when read, returns a
* different set of fields from the ones you wrote, as is common with
* many configuration endpoints. Defaults to false.
*
*/
public Output> ignoreAbsentFields() {
return Codegen.optional(this.ignoreAbsentFields);
}
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
* The full logical path at which to write the given
* data. Consult each backend's documentation to see which endpoints
* support the `PUT` methods and to determine whether they also support
* `DELETE` and `GET`.
*
*/
@Export(name="path", refs={String.class}, tree="[0]")
private Output path;
/**
* @return The full logical path at which to write the given
* data. Consult each backend's documentation to see which endpoints
* support the `PUT` methods and to determine whether they also support
* `DELETE` and `GET`.
*
*/
public Output path() {
return this.path;
}
/**
* - A map whose keys are the top-level data keys
* returned from Vault by the write operation and whose values are the
* corresponding values. This map can only represent string data, so
* any non-string values returned from Vault are serialized as JSON.
* Only fields set in `write_fields` are present in the JSON data.
*
*/
@Export(name="writeData", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy