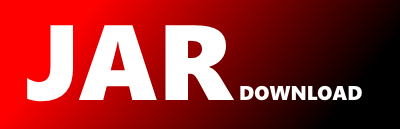
com.pulumi.vault.identity.inputs.GroupPoliciesState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.identity.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GroupPoliciesState extends com.pulumi.resources.ResourceArgs {
public static final GroupPoliciesState Empty = new GroupPoliciesState();
/**
* Defaults to `true`.
*
* If `true`, this resource will take exclusive control of the policies assigned to the group and will set it equal to what is specified in the resource.
*
* If set to `false`, this resource will simply ensure that the policies specified in the resource are present in the group. When destroying the resource, the resource will ensure that the policies specified in the resource are removed.
*
*/
@Import(name="exclusive")
private @Nullable Output exclusive;
/**
* @return Defaults to `true`.
*
* If `true`, this resource will take exclusive control of the policies assigned to the group and will set it equal to what is specified in the resource.
*
* If set to `false`, this resource will simply ensure that the policies specified in the resource are present in the group. When destroying the resource, the resource will ensure that the policies specified in the resource are removed.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy