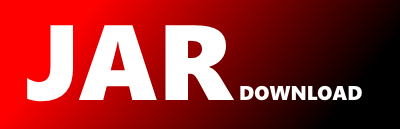
com.pulumi.vault.kubernetes.inputs.GetAuthBackendConfigArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.kubernetes.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetAuthBackendConfigArgs extends com.pulumi.resources.InvokeArgs {
public static final GetAuthBackendConfigArgs Empty = new GetAuthBackendConfigArgs();
/**
* The unique name for the Kubernetes backend the config to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
*
*/
@Import(name="backend")
private @Nullable Output backend;
/**
* @return The unique name for the Kubernetes backend the config to
* retrieve Role attributes for resides in. Defaults to "kubernetes".
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy