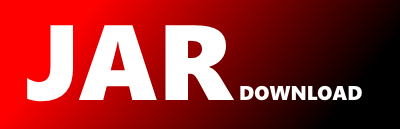
com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.pkiSecret;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.vault.Utilities;
import com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSignedArgs;
import com.pulumi.vault.pkiSecret.inputs.SecretBackendIntermediateSetSignedState;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.Mount;
* import com.pulumi.vault.MountArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendRootCert;
* import com.pulumi.vault.pkiSecret.SecretBackendRootCertArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateCertRequest;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateCertRequestArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendRootSignIntermediate;
* import com.pulumi.vault.pkiSecret.SecretBackendRootSignIntermediateArgs;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSigned;
* import com.pulumi.vault.pkiSecret.SecretBackendIntermediateSetSignedArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var root = new Mount("root", MountArgs.builder()
* .path("pki-root")
* .type("pki")
* .description("root")
* .defaultLeaseTtlSeconds(8640000)
* .maxLeaseTtlSeconds(8640000)
* .build());
*
* var intermediate = new Mount("intermediate", MountArgs.builder()
* .path("pki-int")
* .type(root.type())
* .description("intermediate")
* .defaultLeaseTtlSeconds(86400)
* .maxLeaseTtlSeconds(86400)
* .build());
*
* var example = new SecretBackendRootCert("example", SecretBackendRootCertArgs.builder()
* .backend(root.path())
* .type("internal")
* .commonName("RootOrg Root CA")
* .ttl(86400)
* .format("pem")
* .privateKeyFormat("der")
* .keyType("rsa")
* .keyBits(4096)
* .excludeCnFromSans(true)
* .ou("Organizational Unit")
* .organization("RootOrg")
* .country("US")
* .locality("San Francisco")
* .province("CA")
* .build());
*
* var exampleSecretBackendIntermediateCertRequest = new SecretBackendIntermediateCertRequest("exampleSecretBackendIntermediateCertRequest", SecretBackendIntermediateCertRequestArgs.builder()
* .backend(intermediate.path())
* .type(example.type())
* .commonName("SubOrg Intermediate CA")
* .build());
*
* var exampleSecretBackendRootSignIntermediate = new SecretBackendRootSignIntermediate("exampleSecretBackendRootSignIntermediate", SecretBackendRootSignIntermediateArgs.builder()
* .backend(root.path())
* .csr(exampleSecretBackendIntermediateCertRequest.csr())
* .commonName("SubOrg Intermediate CA")
* .excludeCnFromSans(true)
* .ou("SubUnit")
* .organization("SubOrg")
* .country("US")
* .locality("San Francisco")
* .province("CA")
* .revoke(true)
* .build());
*
* var exampleSecretBackendIntermediateSetSigned = new SecretBackendIntermediateSetSigned("exampleSecretBackendIntermediateSetSigned", SecretBackendIntermediateSetSignedArgs.builder()
* .backend(intermediate.path())
* .certificate(exampleSecretBackendRootSignIntermediate.certificate())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="vault:pkiSecret/secretBackendIntermediateSetSigned:SecretBackendIntermediateSetSigned")
public class SecretBackendIntermediateSetSigned extends com.pulumi.resources.CustomResource {
/**
* The PKI secret backend the resource belongs to.
*
*/
@Export(name="backend", refs={String.class}, tree="[0]")
private Output backend;
/**
* @return The PKI secret backend the resource belongs to.
*
*/
public Output backend() {
return this.backend;
}
/**
* Specifies the PEM encoded certificate. May optionally append additional
* CA certificates to populate the whole chain, which will then enable returning the full chain from
* issue and sign operations.
*
*/
@Export(name="certificate", refs={String.class}, tree="[0]")
private Output certificate;
/**
* @return Specifies the PEM encoded certificate. May optionally append additional
* CA certificates to populate the whole chain, which will then enable returning the full chain from
* issue and sign operations.
*
*/
public Output certificate() {
return this.certificate;
}
/**
* The imported issuers indicating which issuers were created as part of
* this request.
*
*/
@Export(name="importedIssuers", refs={List.class,String.class}, tree="[0,1]")
private Output> importedIssuers;
/**
* @return The imported issuers indicating which issuers were created as part of
* this request.
*
*/
public Output> importedIssuers() {
return this.importedIssuers;
}
/**
* The imported keys indicating which keys were created as part of this request.
*
*/
@Export(name="importedKeys", refs={List.class,String.class}, tree="[0,1]")
private Output> importedKeys;
/**
* @return The imported keys indicating which keys were created as part of this request.
*
*/
public Output> importedKeys() {
return this.importedKeys;
}
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public SecretBackendIntermediateSetSigned(java.lang.String name) {
this(name, SecretBackendIntermediateSetSignedArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public SecretBackendIntermediateSetSigned(java.lang.String name, SecretBackendIntermediateSetSignedArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public SecretBackendIntermediateSetSigned(java.lang.String name, SecretBackendIntermediateSetSignedArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vault:pkiSecret/secretBackendIntermediateSetSigned:SecretBackendIntermediateSetSigned", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private SecretBackendIntermediateSetSigned(java.lang.String name, Output id, @Nullable SecretBackendIntermediateSetSignedState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vault:pkiSecret/secretBackendIntermediateSetSigned:SecretBackendIntermediateSetSigned", name, state, makeResourceOptions(options, id), false);
}
private static SecretBackendIntermediateSetSignedArgs makeArgs(SecretBackendIntermediateSetSignedArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SecretBackendIntermediateSetSignedArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static SecretBackendIntermediateSetSigned get(java.lang.String name, Output id, @Nullable SecretBackendIntermediateSetSignedState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new SecretBackendIntermediateSetSigned(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy