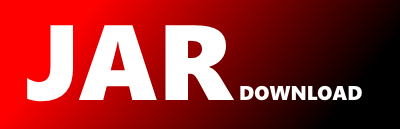
com.pulumi.vault.secrets.SyncGcpDestinationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.secrets;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SyncGcpDestinationArgs extends com.pulumi.resources.ResourceArgs {
public static final SyncGcpDestinationArgs Empty = new SyncGcpDestinationArgs();
/**
* JSON-encoded credentials to use to connect to GCP.
* Can be omitted and directly provided to Vault using the `GOOGLE_APPLICATION_CREDENTIALS` environment
* variable.
*
*/
@Import(name="credentials")
private @Nullable Output credentials;
/**
* @return JSON-encoded credentials to use to connect to GCP.
* Can be omitted and directly provided to Vault using the `GOOGLE_APPLICATION_CREDENTIALS` environment
* variable.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy