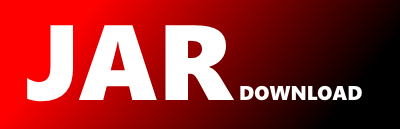
com.pulumi.vault.secrets.SyncGhDestinationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vault Show documentation
Show all versions of vault Show documentation
A Pulumi package for creating and managing HashiCorp Vault cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vault.secrets;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SyncGhDestinationArgs extends com.pulumi.resources.ResourceArgs {
public static final SyncGhDestinationArgs Empty = new SyncGhDestinationArgs();
/**
* Fine-grained or personal access token.
* Can be omitted and directly provided to Vault using the `GITHUB_ACCESS_TOKEN` environment
* variable.
*
*/
@Import(name="accessToken")
private @Nullable Output accessToken;
/**
* @return Fine-grained or personal access token.
* Can be omitted and directly provided to Vault using the `GITHUB_ACCESS_TOKEN` environment
* variable.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy