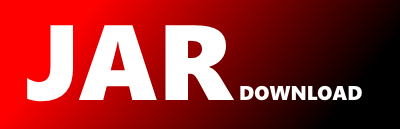
com.pulumi.venafi.Certificate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of venafi Show documentation
Show all versions of venafi Show documentation
A Pulumi package for creating and managing venafi cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.venafi;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.venafi.CertificateArgs;
import com.pulumi.venafi.Utilities;
import com.pulumi.venafi.inputs.CertificateState;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
@ResourceType(type="venafi:index/certificate:Certificate")
public class Certificate extends com.pulumi.resources.CustomResource {
/**
* Key encryption algorithm, either RSA or ECDSA. Defaults to `RSA`.
*
*/
@Export(name="algorithm", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> algorithm;
/**
* @return Key encryption algorithm, either RSA or ECDSA. Defaults to `RSA`.
*
*/
public Output> algorithm() {
return Codegen.optional(this.algorithm);
}
/**
* The X509 certificate in PEM format.
*
*/
@Export(name="certificate", refs={String.class}, tree="[0]")
private Output certificate;
/**
* @return The X509 certificate in PEM format.
*
*/
public Output certificate() {
return this.certificate;
}
@Export(name="certificateDn", refs={String.class}, tree="[0]")
private Output certificateDn;
public Output certificateDn() {
return this.certificateDn;
}
/**
* ID of the issued certificate
*
*/
@Export(name="certificateId", refs={String.class}, tree="[0]")
private Output certificateId;
/**
* @return ID of the issued certificate
*
*/
public Output certificateId() {
return this.certificateId;
}
/**
* The trust chain of X509 certificate authority certificates in PEM format concatenated together.
*
*/
@Export(name="chain", refs={String.class}, tree="[0]")
private Output chain;
/**
* @return The trust chain of X509 certificate authority certificates in PEM format concatenated together.
*
*/
public Output chain() {
return this.chain;
}
/**
* The common name of the certificate.
*
*/
@Export(name="commonName", refs={String.class}, tree="[0]")
private Output commonName;
/**
* @return The common name of the certificate.
*
*/
public Output commonName() {
return this.commonName;
}
/**
* Country of the certificate (C)
*
*/
@Export(name="country", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> country;
/**
* @return Country of the certificate (C)
*
*/
public Output> country() {
return Codegen.optional(this.country);
}
/**
* Whether key-pair generation will be `local` or `service` generated. Default is
* `local`.
*
*/
@Export(name="csrOrigin", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> csrOrigin;
/**
* @return Whether key-pair generation will be `local` or `service` generated. Default is
* `local`.
*
*/
public Output> csrOrigin() {
return Codegen.optional(this.csrOrigin);
}
@Export(name="csrPem", refs={String.class}, tree="[0]")
private Output csrPem;
public Output csrPem() {
return this.csrPem;
}
/**
* Collection of Custom Field name-value pairs to assign to the certificate.
*
*/
@Export(name="customFields", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> customFields;
/**
* @return Collection of Custom Field name-value pairs to assign to the certificate.
*
*/
public Output>> customFields() {
return Codegen.optional(this.customFields);
}
/**
* ECDSA curve to use when generating a key
*
*/
@Export(name="ecdsaCurve", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ecdsaCurve;
/**
* @return ECDSA curve to use when generating a key
*
*/
public Output> ecdsaCurve() {
return Codegen.optional(this.ecdsaCurve);
}
/**
* Number of hours before certificate expiry to request a new certificate.
* Defaults to `168`.
*
*/
@Export(name="expirationWindow", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> expirationWindow;
/**
* @return Number of hours before certificate expiry to request a new certificate.
* Defaults to `168`.
*
*/
public Output> expirationWindow() {
return Codegen.optional(this.expirationWindow);
}
/**
* Used with `valid_days` to indicate the target issuer when using Trust Protection
* Platform. Relevant values are: `DigiCert`, `Entrust`, and `Microsoft`.
*
*/
@Export(name="issuerHint", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> issuerHint;
/**
* @return Used with `valid_days` to indicate the target issuer when using Trust Protection
* Platform. Relevant values are: `DigiCert`, `Entrust`, and `Microsoft`.
*
*/
public Output> issuerHint() {
return Codegen.optional(this.issuerHint);
}
/**
* The password used to encrypt the private key.
*
*/
@Export(name="keyPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> keyPassword;
/**
* @return The password used to encrypt the private key.
*
*/
public Output> keyPassword() {
return Codegen.optional(this.keyPassword);
}
/**
* Locality/City of the certificate (L)
*
*/
@Export(name="locality", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> locality;
/**
* @return Locality/City of the certificate (L)
*
*/
public Output> locality() {
return Codegen.optional(this.locality);
}
/**
* Use to specify a name for the new certificate object that will be created and placed
* in a policy. Only valid for Trust Protection Platform.
*
*/
@Export(name="nickname", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> nickname;
/**
* @return Use to specify a name for the new certificate object that will be created and placed
* in a policy. Only valid for Trust Protection Platform.
*
*/
public Output> nickname() {
return Codegen.optional(this.nickname);
}
/**
* Organization of the certificate (O)
*
*/
@Export(name="organization", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> organization;
/**
* @return Organization of the certificate (O)
*
*/
public Output> organization() {
return Codegen.optional(this.organization);
}
/**
* List of Organizational Units of the certificate (OU)
*
*/
@Export(name="organizationalUnits", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> organizationalUnits;
/**
* @return List of Organizational Units of the certificate (OU)
*
*/
public Output>> organizationalUnits() {
return Codegen.optional(this.organizationalUnits);
}
/**
* A base64-encoded PKCS#12 keystore secured by the `key_password`. Useful when working with resources like
* azure key_vault_certificate.
*
*/
@Export(name="pkcs12", refs={String.class}, tree="[0]")
private Output pkcs12;
/**
* @return A base64-encoded PKCS#12 keystore secured by the `key_password`. Useful when working with resources like
* azure key_vault_certificate.
*
*/
public Output pkcs12() {
return this.pkcs12;
}
/**
* The private key in PEM format.
*
*/
@Export(name="privateKeyPem", refs={String.class}, tree="[0]")
private Output privateKeyPem;
/**
* @return The private key in PEM format.
*
*/
public Output privateKeyPem() {
return this.privateKeyPem;
}
/**
* Indicates the certificate should be reissued. This means the resource will destroyed and recreated
*
*/
@Export(name="renewRequired", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> renewRequired;
/**
* @return Indicates the certificate should be reissued. This means the resource will destroyed and recreated
*
*/
public Output> renewRequired() {
return Codegen.optional(this.renewRequired);
}
/**
* Number of bits to use when generating an RSA key. Applies when algorithm is `RSA`.
* Defaults to `2048`.
*
*/
@Export(name="rsaBits", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> rsaBits;
/**
* @return Number of bits to use when generating an RSA key. Applies when algorithm is `RSA`.
* Defaults to `2048`.
*
*/
public Output> rsaBits() {
return Codegen.optional(this.rsaBits);
}
/**
* List of DNS names to use as alternative subjects of the certificate.
*
*/
@Export(name="sanDns", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sanDns;
/**
* @return List of DNS names to use as alternative subjects of the certificate.
*
*/
public Output>> sanDns() {
return Codegen.optional(this.sanDns);
}
/**
* List of email addresses to use as alternative subjects of the certificate.
*
*/
@Export(name="sanEmails", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sanEmails;
/**
* @return List of email addresses to use as alternative subjects of the certificate.
*
*/
public Output>> sanEmails() {
return Codegen.optional(this.sanEmails);
}
/**
* List of IP addresses to use as alternative subjects of the certificate.
*
*/
@Export(name="sanIps", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sanIps;
/**
* @return List of IP addresses to use as alternative subjects of the certificate.
*
*/
public Output>> sanIps() {
return Codegen.optional(this.sanIps);
}
/**
* List of Uniform Resource Identifiers (URIs) to use as alternative subjects of
* the certificate.
*
*/
@Export(name="sanUris", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> sanUris;
/**
* @return List of Uniform Resource Identifiers (URIs) to use as alternative subjects of
* the certificate.
*
*/
public Output>> sanUris() {
return Codegen.optional(this.sanUris);
}
/**
* State of the certificate (S)
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> state;
/**
* @return State of the certificate (S)
*
*/
public Output> state() {
return Codegen.optional(this.state);
}
/**
* Desired number of days for which the new certificate will be valid.
*
*/
@Export(name="validDays", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> validDays;
/**
* @return Desired number of days for which the new certificate will be valid.
*
*/
public Output> validDays() {
return Codegen.optional(this.validDays);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Certificate(java.lang.String name) {
this(name, CertificateArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Certificate(java.lang.String name, CertificateArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Certificate(java.lang.String name, CertificateArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("venafi:index/certificate:Certificate", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Certificate(java.lang.String name, Output id, @Nullable CertificateState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("venafi:index/certificate:Certificate", name, state, makeResourceOptions(options, id), false);
}
private static CertificateArgs makeArgs(CertificateArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? CertificateArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"keyPassword",
"privateKeyPem"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Certificate get(java.lang.String name, Output id, @Nullable CertificateState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Certificate(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy