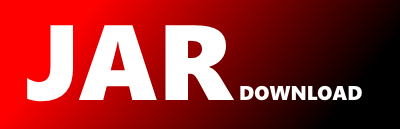
com.pulumi.venafi.inputs.CertificateState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of venafi Show documentation
Show all versions of venafi Show documentation
A Pulumi package for creating and managing venafi cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.venafi.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CertificateState extends com.pulumi.resources.ResourceArgs {
public static final CertificateState Empty = new CertificateState();
/**
* Key encryption algorithm, either RSA or ECDSA. Defaults to `RSA`.
*
*/
@Import(name="algorithm")
private @Nullable Output algorithm;
/**
* @return Key encryption algorithm, either RSA or ECDSA. Defaults to `RSA`.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy