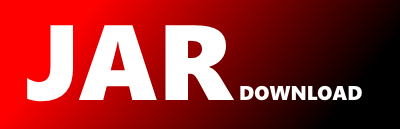
com.pulumi.vsphere.StorageDrsVmOverrideArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StorageDrsVmOverrideArgs extends com.pulumi.resources.ResourceArgs {
public static final StorageDrsVmOverrideArgs Empty = new StorageDrsVmOverrideArgs();
/**
* The managed object reference
* ID of the datastore cluster to put the override in.
* Forces a new resource if changed.
*
*/
@Import(name="datastoreClusterId", required=true)
private Output datastoreClusterId;
/**
* @return The managed object reference
* ID of the datastore cluster to put the override in.
* Forces a new resource if changed.
*
*/
public Output datastoreClusterId() {
return this.datastoreClusterId;
}
/**
* Overrides any Storage DRS automation
* levels for this virtual machine. Can be one of `automated` or `manual`. When
* not specified, the datastore cluster's settings are used according to the
* specific SDRS subsystem.
*
*/
@Import(name="sdrsAutomationLevel")
private @Nullable Output sdrsAutomationLevel;
/**
* @return Overrides any Storage DRS automation
* levels for this virtual machine. Can be one of `automated` or `manual`. When
* not specified, the datastore cluster's settings are used according to the
* specific SDRS subsystem.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy