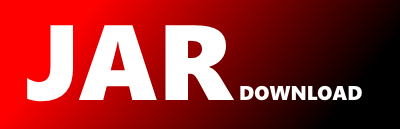
com.pulumi.vsphere.Vnic Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.vsphere.Utilities;
import com.pulumi.vsphere.VnicArgs;
import com.pulumi.vsphere.inputs.VnicState;
import com.pulumi.vsphere.outputs.VnicIpv4;
import com.pulumi.vsphere.outputs.VnicIpv6;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a VMware vSphere vnic resource.
*
* ## Example Usage
*
* ### S
* ### Create a vnic attached to a distributed virtual switch using the vmotion TCP/IP stack
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vsphere.VsphereFunctions;
* import com.pulumi.vsphere.inputs.GetDatacenterArgs;
* import com.pulumi.vsphere.inputs.GetHostArgs;
* import com.pulumi.vsphere.DistributedVirtualSwitch;
* import com.pulumi.vsphere.DistributedVirtualSwitchArgs;
* import com.pulumi.vsphere.inputs.DistributedVirtualSwitchHostArgs;
* import com.pulumi.vsphere.DistributedPortGroup;
* import com.pulumi.vsphere.DistributedPortGroupArgs;
* import com.pulumi.vsphere.Vnic;
* import com.pulumi.vsphere.VnicArgs;
* import com.pulumi.vsphere.inputs.VnicIpv4Args;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var dc = VsphereFunctions.getDatacenter(GetDatacenterArgs.builder()
* .name("mydc")
* .build());
*
* final var h1 = VsphereFunctions.getHost(GetHostArgs.builder()
* .name("esxi1.host.test")
* .datacenterId(dc.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* var d1 = new DistributedVirtualSwitch("d1", DistributedVirtualSwitchArgs.builder()
* .datacenterId(dc.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .hosts(DistributedVirtualSwitchHostArgs.builder()
* .hostSystemId(h1.applyValue(getHostResult -> getHostResult.id()))
* .devices("vnic3")
* .build())
* .build());
*
* var p1 = new DistributedPortGroup("p1", DistributedPortGroupArgs.builder()
* .vlanId(1234)
* .distributedVirtualSwitchUuid(d1.id())
* .build());
*
* var v1 = new Vnic("v1", VnicArgs.builder()
* .host(h1.applyValue(getHostResult -> getHostResult.id()))
* .distributedSwitchPort(d1.id())
* .distributedPortGroup(p1.id())
* .ipv4(VnicIpv4Args.builder()
* .dhcp(true)
* .build())
* .netstack("vmotion")
* .build());
*
* }
* }
* ```
* ### Create a vnic attached to a portgroup using the default TCP/IP stack
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vsphere.VsphereFunctions;
* import com.pulumi.vsphere.inputs.GetDatacenterArgs;
* import com.pulumi.vsphere.inputs.GetHostArgs;
* import com.pulumi.vsphere.HostVirtualSwitch;
* import com.pulumi.vsphere.HostVirtualSwitchArgs;
* import com.pulumi.vsphere.HostPortGroup;
* import com.pulumi.vsphere.HostPortGroupArgs;
* import com.pulumi.vsphere.Vnic;
* import com.pulumi.vsphere.VnicArgs;
* import com.pulumi.vsphere.inputs.VnicIpv4Args;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var dc = VsphereFunctions.getDatacenter(GetDatacenterArgs.builder()
* .name("mydc")
* .build());
*
* final var h1 = VsphereFunctions.getHost(GetHostArgs.builder()
* .name("esxi1.host.test")
* .datacenterId(dc.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* var hvs1 = new HostVirtualSwitch("hvs1", HostVirtualSwitchArgs.builder()
* .hostSystemId(h1.applyValue(getHostResult -> getHostResult.id()))
* .networkAdapters(
* "vmnic3",
* "vmnic4")
* .activeNics("vmnic3")
* .standbyNics("vmnic4")
* .build());
*
* var p1 = new HostPortGroup("p1", HostPortGroupArgs.builder()
* .virtualSwitchName(hvs1.name())
* .hostSystemId(h1.applyValue(getHostResult -> getHostResult.id()))
* .build());
*
* var v1 = new Vnic("v1", VnicArgs.builder()
* .host(h1.applyValue(getHostResult -> getHostResult.id()))
* .portgroup(p1.name())
* .ipv4(VnicIpv4Args.builder()
* .dhcp(true)
* .build())
* .services(
* "vsan",
* "management")
* .build());
*
* }
* }
* ```
* ## Importing
*
* An existing vNic can be [imported][docs-import] into this resource
* via supplying the vNic's ID. An example is below:
*
* [docs-import]: /docs/import/index.html
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* }
* }
* ```
*
* The above would import the vnic `vmk2` from host with ID `host-123`.
*
*/
@ResourceType(type="vsphere:index/vnic:Vnic")
public class Vnic extends com.pulumi.resources.CustomResource {
/**
* Key of the distributed portgroup the nic will connect to.
*
*/
@Export(name="distributedPortGroup", type=String.class, parameters={})
private Output* @Nullable */ String> distributedPortGroup;
/**
* @return Key of the distributed portgroup the nic will connect to.
*
*/
public Output> distributedPortGroup() {
return Codegen.optional(this.distributedPortGroup);
}
/**
* UUID of the DVSwitch the nic will be attached to. Do not set if you set portgroup.
*
*/
@Export(name="distributedSwitchPort", type=String.class, parameters={})
private Output* @Nullable */ String> distributedSwitchPort;
/**
* @return UUID of the DVSwitch the nic will be attached to. Do not set if you set portgroup.
*
*/
public Output> distributedSwitchPort() {
return Codegen.optional(this.distributedSwitchPort);
}
/**
* ESX host the interface belongs to
*
*/
@Export(name="host", type=String.class, parameters={})
private Output host;
/**
* @return ESX host the interface belongs to
*
*/
public Output host() {
return this.host;
}
/**
* IPv4 settings. Either this or `ipv6` needs to be set. See IPv4 options below.
*
*/
@Export(name="ipv4", type=VnicIpv4.class, parameters={})
private Output* @Nullable */ VnicIpv4> ipv4;
/**
* @return IPv4 settings. Either this or `ipv6` needs to be set. See IPv4 options below.
*
*/
public Output> ipv4() {
return Codegen.optional(this.ipv4);
}
/**
* IPv6 settings. Either this or `ipv6` needs to be set. See IPv6 options below.
*
*/
@Export(name="ipv6", type=VnicIpv6.class, parameters={})
private Output* @Nullable */ VnicIpv6> ipv6;
/**
* @return IPv6 settings. Either this or `ipv6` needs to be set. See IPv6 options below.
*
*/
public Output> ipv6() {
return Codegen.optional(this.ipv6);
}
/**
* MAC address of the interface.
*
*/
@Export(name="mac", type=String.class, parameters={})
private Output mac;
/**
* @return MAC address of the interface.
*
*/
public Output mac() {
return this.mac;
}
/**
* MTU of the interface.
*
*/
@Export(name="mtu", type=Integer.class, parameters={})
private Output mtu;
/**
* @return MTU of the interface.
*
*/
public Output mtu() {
return this.mtu;
}
/**
* TCP/IP stack setting for this interface. Possible values are `defaultTcpipStack``, 'vmotion', 'vSphereProvisioning'. Changing this will force the creation of a new interface since it's not possible to change the stack once it gets created. (Default:`defaultTcpipStack`)
*
*/
@Export(name="netstack", type=String.class, parameters={})
private Output* @Nullable */ String> netstack;
/**
* @return TCP/IP stack setting for this interface. Possible values are `defaultTcpipStack``, 'vmotion', 'vSphereProvisioning'. Changing this will force the creation of a new interface since it's not possible to change the stack once it gets created. (Default:`defaultTcpipStack`)
*
*/
public Output> netstack() {
return Codegen.optional(this.netstack);
}
/**
* Portgroup to attach the nic to. Do not set if you set distributed_switch_port.
*
*/
@Export(name="portgroup", type=String.class, parameters={})
private Output* @Nullable */ String> portgroup;
/**
* @return Portgroup to attach the nic to. Do not set if you set distributed_switch_port.
*
*/
public Output> portgroup() {
return Codegen.optional(this.portgroup);
}
/**
* Enabled services setting for this interface. Currently support values are `vmotion`, `management`, and `vsan`.
*
*/
@Export(name="services", type=List.class, parameters={String.class})
private Output* @Nullable */ List> services;
/**
* @return Enabled services setting for this interface. Currently support values are `vmotion`, `management`, and `vsan`.
*
*/
public Output>> services() {
return Codegen.optional(this.services);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Vnic(String name) {
this(name, VnicArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Vnic(String name, VnicArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Vnic(String name, VnicArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/vnic:Vnic", name, args == null ? VnicArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private Vnic(String name, Output id, @Nullable VnicState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/vnic:Vnic", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Vnic get(String name, Output id, @Nullable VnicState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Vnic(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy