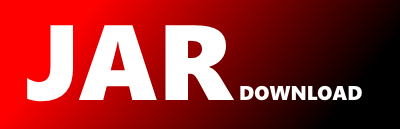
com.pulumi.vsphere.ComputeClusterVmAffinityRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.vsphere.ComputeClusterVmAffinityRuleArgs;
import com.pulumi.vsphere.Utilities;
import com.pulumi.vsphere.inputs.ComputeClusterVmAffinityRuleState;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The `vsphere.ComputeClusterVmAffinityRule` resource can be used to
* manage virtual machine affinity rules in a cluster, either created by the
* `vsphere.ComputeCluster` resource or looked up
* by the `vsphere.ComputeCluster` data source.
*
* This rule can be used to tell a set of virtual machines to run together on the
* same host within a cluster. When configured, DRS will make a best effort to
* ensure that the virtual machines run on the same host, or prevent any operation
* that would keep that from happening, depending on the value of the
* `mandatory` flag.
*
* > An affinity rule can only be used to place virtual machines on the same
* _non-specific_ hosts. It cannot be used to pin virtual machines to a host.
* To enable this capability, use the
* `vsphere.ComputeClusterVmHostRule`
* resource.
*
* > **NOTE:** This resource requires vCenter Server and is not available on
* direct ESXi host connections.
*
* ## Example Usage
*
* The following example creates two virtual machines in a cluster using the
* `vsphere.VirtualMachine` resource, creating the
* virtual machines in the cluster looked up by the
* `vsphere.ComputeCluster` data source. It
* then creates an affinity rule for these two virtual machines, ensuring they
* will run on the same host whenever possible.
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* The following example creates an affinity rule for a set of virtual machines
* in the cluster by looking up the virtual machine UUIDs from the
* `vsphere.VirtualMachine` data source.
*
* <!--Start PulumiCodeChooser -->
* <!--End PulumiCodeChooser -->
*
* ## Importing
*
* An existing rule can be imported into this resource by supplying
* both the path to the cluster, and the name the rule. If the name or cluster is
* not found, or if the rule is of a different type, an error will be returned. An
* example is below:
*
*/
@ResourceType(type="vsphere:index/computeClusterVmAffinityRule:ComputeClusterVmAffinityRule")
public class ComputeClusterVmAffinityRule extends com.pulumi.resources.CustomResource {
/**
* The managed object reference
* ID of the cluster to put the group in. Forces a new
* resource if changed.
*
*/
@Export(name="computeClusterId", refs={String.class}, tree="[0]")
private Output computeClusterId;
/**
* @return The managed object reference
* ID of the cluster to put the group in. Forces a new
* resource if changed.
*
*/
public Output computeClusterId() {
return this.computeClusterId;
}
/**
* Enable this rule in the cluster. Default: `true`.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enabled;
/**
* @return Enable this rule in the cluster. Default: `true`.
*
*/
public Output> enabled() {
return Codegen.optional(this.enabled);
}
/**
* When this value is `true`, prevents any virtual
* machine operations that may violate this rule. Default: `false`.
*
* > **NOTE:** The namespace for rule names on this resource (defined by the
* `name` argument) is shared with all rules in the cluster - consider
* this when naming your rules.
*
*/
@Export(name="mandatory", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> mandatory;
/**
* @return When this value is `true`, prevents any virtual
* machine operations that may violate this rule. Default: `false`.
*
* > **NOTE:** The namespace for rule names on this resource (defined by the
* `name` argument) is shared with all rules in the cluster - consider
* this when naming your rules.
*
*/
public Output> mandatory() {
return Codegen.optional(this.mandatory);
}
/**
* The name of the rule. This must be unique in the cluster.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the rule. This must be unique in the cluster.
*
*/
public Output name() {
return this.name;
}
/**
* The UUIDs of the virtual machines to run
* on the same host together.
*
*/
@Export(name="virtualMachineIds", refs={List.class,String.class}, tree="[0,1]")
private Output> virtualMachineIds;
/**
* @return The UUIDs of the virtual machines to run
* on the same host together.
*
*/
public Output> virtualMachineIds() {
return this.virtualMachineIds;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ComputeClusterVmAffinityRule(java.lang.String name) {
this(name, ComputeClusterVmAffinityRuleArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ComputeClusterVmAffinityRule(java.lang.String name, ComputeClusterVmAffinityRuleArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ComputeClusterVmAffinityRule(java.lang.String name, ComputeClusterVmAffinityRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/computeClusterVmAffinityRule:ComputeClusterVmAffinityRule", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ComputeClusterVmAffinityRule(java.lang.String name, Output id, @Nullable ComputeClusterVmAffinityRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/computeClusterVmAffinityRule:ComputeClusterVmAffinityRule", name, state, makeResourceOptions(options, id), false);
}
private static ComputeClusterVmAffinityRuleArgs makeArgs(ComputeClusterVmAffinityRuleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ComputeClusterVmAffinityRuleArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ComputeClusterVmAffinityRule get(java.lang.String name, Output id, @Nullable ComputeClusterVmAffinityRuleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ComputeClusterVmAffinityRule(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy