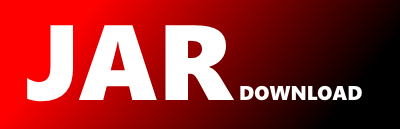
com.pulumi.vsphere.VirtualMachineClassArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualMachineClassArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachineClassArgs Empty = new VirtualMachineClassArgs();
/**
* The percentage of the available CPU capacity which will be reserved.
*
*/
@Import(name="cpuReservation")
private @Nullable Output cpuReservation;
/**
* @return The percentage of the available CPU capacity which will be reserved.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy