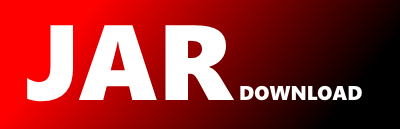
com.pulumi.vsphere.outputs.GetGuestOsCustomizationSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.vsphere.outputs.GetGuestOsCustomizationSpecLinuxOption;
import com.pulumi.vsphere.outputs.GetGuestOsCustomizationSpecNetworkInterface;
import com.pulumi.vsphere.outputs.GetGuestOsCustomizationSpecWindowsOption;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetGuestOsCustomizationSpec {
/**
* @return A list of DNS servers for a virtual network adapter with a static IP address.
*
*/
private List dnsServerLists;
/**
* @return A list of DNS search domains to add to the DNS configuration on the virtual machine.
*
*/
private List dnsSuffixLists;
/**
* @return A list of configuration options specific to Linux.
*
*/
private List linuxOptions;
/**
* @return A specification of network interface configuration options.
*
*/
private List networkInterfaces;
/**
* @return A list of configuration options specific to Windows.
*
*/
private List windowsOptions;
/**
* @return Use this option to specify use of a Windows Sysprep file.
*
*/
private String windowsSysprepText;
private GetGuestOsCustomizationSpec() {}
/**
* @return A list of DNS servers for a virtual network adapter with a static IP address.
*
*/
public List dnsServerLists() {
return this.dnsServerLists;
}
/**
* @return A list of DNS search domains to add to the DNS configuration on the virtual machine.
*
*/
public List dnsSuffixLists() {
return this.dnsSuffixLists;
}
/**
* @return A list of configuration options specific to Linux.
*
*/
public List linuxOptions() {
return this.linuxOptions;
}
/**
* @return A specification of network interface configuration options.
*
*/
public List networkInterfaces() {
return this.networkInterfaces;
}
/**
* @return A list of configuration options specific to Windows.
*
*/
public List windowsOptions() {
return this.windowsOptions;
}
/**
* @return Use this option to specify use of a Windows Sysprep file.
*
*/
public String windowsSysprepText() {
return this.windowsSysprepText;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetGuestOsCustomizationSpec defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List dnsServerLists;
private List dnsSuffixLists;
private List linuxOptions;
private List networkInterfaces;
private List windowsOptions;
private String windowsSysprepText;
public Builder() {}
public Builder(GetGuestOsCustomizationSpec defaults) {
Objects.requireNonNull(defaults);
this.dnsServerLists = defaults.dnsServerLists;
this.dnsSuffixLists = defaults.dnsSuffixLists;
this.linuxOptions = defaults.linuxOptions;
this.networkInterfaces = defaults.networkInterfaces;
this.windowsOptions = defaults.windowsOptions;
this.windowsSysprepText = defaults.windowsSysprepText;
}
@CustomType.Setter
public Builder dnsServerLists(List dnsServerLists) {
if (dnsServerLists == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "dnsServerLists");
}
this.dnsServerLists = dnsServerLists;
return this;
}
public Builder dnsServerLists(String... dnsServerLists) {
return dnsServerLists(List.of(dnsServerLists));
}
@CustomType.Setter
public Builder dnsSuffixLists(List dnsSuffixLists) {
if (dnsSuffixLists == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "dnsSuffixLists");
}
this.dnsSuffixLists = dnsSuffixLists;
return this;
}
public Builder dnsSuffixLists(String... dnsSuffixLists) {
return dnsSuffixLists(List.of(dnsSuffixLists));
}
@CustomType.Setter
public Builder linuxOptions(List linuxOptions) {
if (linuxOptions == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "linuxOptions");
}
this.linuxOptions = linuxOptions;
return this;
}
public Builder linuxOptions(GetGuestOsCustomizationSpecLinuxOption... linuxOptions) {
return linuxOptions(List.of(linuxOptions));
}
@CustomType.Setter
public Builder networkInterfaces(List networkInterfaces) {
if (networkInterfaces == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "networkInterfaces");
}
this.networkInterfaces = networkInterfaces;
return this;
}
public Builder networkInterfaces(GetGuestOsCustomizationSpecNetworkInterface... networkInterfaces) {
return networkInterfaces(List.of(networkInterfaces));
}
@CustomType.Setter
public Builder windowsOptions(List windowsOptions) {
if (windowsOptions == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "windowsOptions");
}
this.windowsOptions = windowsOptions;
return this;
}
public Builder windowsOptions(GetGuestOsCustomizationSpecWindowsOption... windowsOptions) {
return windowsOptions(List.of(windowsOptions));
}
@CustomType.Setter
public Builder windowsSysprepText(String windowsSysprepText) {
if (windowsSysprepText == null) {
throw new MissingRequiredPropertyException("GetGuestOsCustomizationSpec", "windowsSysprepText");
}
this.windowsSysprepText = windowsSysprepText;
return this;
}
public GetGuestOsCustomizationSpec build() {
final var _resultValue = new GetGuestOsCustomizationSpec();
_resultValue.dnsServerLists = dnsServerLists;
_resultValue.dnsSuffixLists = dnsSuffixLists;
_resultValue.linuxOptions = linuxOptions;
_resultValue.networkInterfaces = networkInterfaces;
_resultValue.windowsOptions = windowsOptions;
_resultValue.windowsSysprepText = windowsSysprepText;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy