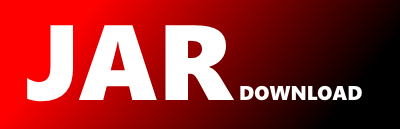
com.pulumi.vsphere.outputs.SupervisorManagementNetwork Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class SupervisorManagementNetwork {
/**
* @return Number of addresses to allocate. Starts from 'starting_address'
*
*/
private Integer addressCount;
/**
* @return Gateway IP address.
*
*/
private String gateway;
/**
* @return ID of the network. (e.g. a distributed port group).
*
*/
private String network;
/**
* @return Starting address of the management network range.
*
*/
private String startingAddress;
/**
* @return Subnet mask.
*
*/
private String subnetMask;
private SupervisorManagementNetwork() {}
/**
* @return Number of addresses to allocate. Starts from 'starting_address'
*
*/
public Integer addressCount() {
return this.addressCount;
}
/**
* @return Gateway IP address.
*
*/
public String gateway() {
return this.gateway;
}
/**
* @return ID of the network. (e.g. a distributed port group).
*
*/
public String network() {
return this.network;
}
/**
* @return Starting address of the management network range.
*
*/
public String startingAddress() {
return this.startingAddress;
}
/**
* @return Subnet mask.
*
*/
public String subnetMask() {
return this.subnetMask;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SupervisorManagementNetwork defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer addressCount;
private String gateway;
private String network;
private String startingAddress;
private String subnetMask;
public Builder() {}
public Builder(SupervisorManagementNetwork defaults) {
Objects.requireNonNull(defaults);
this.addressCount = defaults.addressCount;
this.gateway = defaults.gateway;
this.network = defaults.network;
this.startingAddress = defaults.startingAddress;
this.subnetMask = defaults.subnetMask;
}
@CustomType.Setter
public Builder addressCount(Integer addressCount) {
if (addressCount == null) {
throw new MissingRequiredPropertyException("SupervisorManagementNetwork", "addressCount");
}
this.addressCount = addressCount;
return this;
}
@CustomType.Setter
public Builder gateway(String gateway) {
if (gateway == null) {
throw new MissingRequiredPropertyException("SupervisorManagementNetwork", "gateway");
}
this.gateway = gateway;
return this;
}
@CustomType.Setter
public Builder network(String network) {
if (network == null) {
throw new MissingRequiredPropertyException("SupervisorManagementNetwork", "network");
}
this.network = network;
return this;
}
@CustomType.Setter
public Builder startingAddress(String startingAddress) {
if (startingAddress == null) {
throw new MissingRequiredPropertyException("SupervisorManagementNetwork", "startingAddress");
}
this.startingAddress = startingAddress;
return this;
}
@CustomType.Setter
public Builder subnetMask(String subnetMask) {
if (subnetMask == null) {
throw new MissingRequiredPropertyException("SupervisorManagementNetwork", "subnetMask");
}
this.subnetMask = subnetMask;
return this;
}
public SupervisorManagementNetwork build() {
final var _resultValue = new SupervisorManagementNetwork();
_resultValue.addressCount = addressCount;
_resultValue.gateway = gateway;
_resultValue.network = network;
_resultValue.startingAddress = startingAddress;
_resultValue.subnetMask = subnetMask;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy