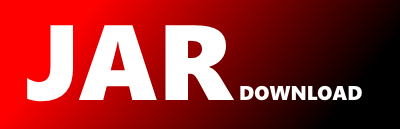
com.pulumi.vsphere.NasDatastoreArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NasDatastoreArgs extends com.pulumi.resources.ResourceArgs {
public static final NasDatastoreArgs Empty = new NasDatastoreArgs();
/**
* Access mode for the mount point. Can be one of
* `readOnly` or `readWrite`. Note that `readWrite` does not necessarily mean
* that the datastore will be read-write depending on the permissions of the
* actual share. Default: `readWrite`. Forces a new resource if changed.
*
*/
@Import(name="accessMode")
private @Nullable Output accessMode;
/**
* @return Access mode for the mount point. Can be one of
* `readOnly` or `readWrite`. Note that `readWrite` does not necessarily mean
* that the datastore will be read-write depending on the permissions of the
* actual share. Default: `readWrite`. Forces a new resource if changed.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy