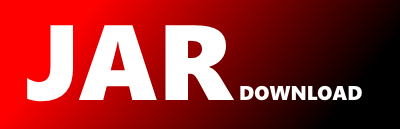
com.pulumi.vsphere.StorageDrsVmOverride Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.vsphere.StorageDrsVmOverrideArgs;
import com.pulumi.vsphere.Utilities;
import com.pulumi.vsphere.inputs.StorageDrsVmOverrideState;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The `vsphere.StorageDrsVmOverride` resource can be used to add a Storage DRS
* override to a datastore cluster for a specific virtual machine. With this
* resource, one can enable or disable Storage DRS, and control the automation
* level and disk affinity for a single virtual machine without affecting the rest
* of the datastore cluster.
*
* For more information on vSphere datastore clusters and Storage DRS, see [this
* page][ref-vsphere-datastore-clusters].
*
* [ref-vsphere-datastore-clusters]: https://docs.vmware.com/en/VMware-vSphere/8.0/vsphere-resource-management/GUID-598DF695-107E-406B-9C95-0AF961FC227A.html
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vsphere.VsphereFunctions;
* import com.pulumi.vsphere.inputs.GetDatacenterArgs;
* import com.pulumi.vsphere.inputs.GetDatastoreClusterArgs;
* import com.pulumi.vsphere.inputs.GetDatastoreArgs;
* import com.pulumi.vsphere.inputs.GetResourcePoolArgs;
* import com.pulumi.vsphere.inputs.GetNetworkArgs;
* import com.pulumi.vsphere.VirtualMachine;
* import com.pulumi.vsphere.VirtualMachineArgs;
* import com.pulumi.vsphere.inputs.VirtualMachineNetworkInterfaceArgs;
* import com.pulumi.vsphere.inputs.VirtualMachineDiskArgs;
* import com.pulumi.vsphere.StorageDrsVmOverride;
* import com.pulumi.vsphere.StorageDrsVmOverrideArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var datacenter = VsphereFunctions.getDatacenter(GetDatacenterArgs.builder()
* .name("dc-01")
* .build());
*
* final var datastoreCluster = VsphereFunctions.getDatastoreCluster(GetDatastoreClusterArgs.builder()
* .name("datastore-cluster1")
* .datacenterId(datacenter.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* final var memberDatastore = VsphereFunctions.getDatastore(GetDatastoreArgs.builder()
* .name("datastore-cluster1-member1")
* .datacenterId(datacenter.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* final var pool = VsphereFunctions.getResourcePool(GetResourcePoolArgs.builder()
* .name("cluster1/Resources")
* .datacenterId(datacenter.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* final var network = VsphereFunctions.getNetwork(GetNetworkArgs.builder()
* .name("public")
* .datacenterId(datacenter.applyValue(getDatacenterResult -> getDatacenterResult.id()))
* .build());
*
* var vm = new VirtualMachine("vm", VirtualMachineArgs.builder()
* .name("test")
* .resourcePoolId(pool.applyValue(getResourcePoolResult -> getResourcePoolResult.id()))
* .datastoreId(memberDatastore.applyValue(getDatastoreResult -> getDatastoreResult.id()))
* .numCpus(2)
* .memory(1024)
* .guestId("otherLinux64Guest")
* .networkInterfaces(VirtualMachineNetworkInterfaceArgs.builder()
* .networkId(network.applyValue(getNetworkResult -> getNetworkResult.id()))
* .build())
* .disks(VirtualMachineDiskArgs.builder()
* .label("disk0")
* .size(20)
* .build())
* .build());
*
* var drsVmOverride = new StorageDrsVmOverride("drsVmOverride", StorageDrsVmOverrideArgs.builder()
* .datastoreClusterId(datastoreCluster.applyValue(getDatastoreClusterResult -> getDatastoreClusterResult.id()))
* .virtualMachineId(vm.id())
* .sdrsEnabled(false)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* An existing override can be imported into this resource by
*
* supplying both the path to the datastore cluster and the path to the virtual
*
* machine to `pulumi import`. If no override exists, an error will be given.
*
* An example is below:
*
* ```sh
* $ pulumi import vsphere:index/storageDrsVmOverride:StorageDrsVmOverride drs_vm_override \
* ```
*
* '{"datastore_cluster_path": "/dc1/datastore/ds-cluster", \
*
* "virtual_machine_path": "/dc1/vm/srv1"}'
*
*/
@ResourceType(type="vsphere:index/storageDrsVmOverride:StorageDrsVmOverride")
public class StorageDrsVmOverride extends com.pulumi.resources.CustomResource {
/**
* The managed object reference
* ID of the datastore cluster to put the override in.
* Forces a new resource if changed.
*
*/
@Export(name="datastoreClusterId", refs={String.class}, tree="[0]")
private Output datastoreClusterId;
/**
* @return The managed object reference
* ID of the datastore cluster to put the override in.
* Forces a new resource if changed.
*
*/
public Output datastoreClusterId() {
return this.datastoreClusterId;
}
/**
* Overrides any Storage DRS automation
* levels for this virtual machine. Can be one of `automated` or `manual`. When
* not specified, the datastore cluster's settings are used according to the
* specific SDRS subsystem.
*
*/
@Export(name="sdrsAutomationLevel", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sdrsAutomationLevel;
/**
* @return Overrides any Storage DRS automation
* levels for this virtual machine. Can be one of `automated` or `manual`. When
* not specified, the datastore cluster's settings are used according to the
* specific SDRS subsystem.
*
*/
public Output> sdrsAutomationLevel() {
return Codegen.optional(this.sdrsAutomationLevel);
}
/**
* Overrides the default Storage DRS setting for
* this virtual machine. When not specified, the datastore cluster setting is
* used.
*
*/
@Export(name="sdrsEnabled", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sdrsEnabled;
/**
* @return Overrides the default Storage DRS setting for
* this virtual machine. When not specified, the datastore cluster setting is
* used.
*
*/
public Output> sdrsEnabled() {
return Codegen.optional(this.sdrsEnabled);
}
/**
* Overrides the intra-VM affinity setting
* for this virtual machine. When `true`, all disks for this virtual machine
* will be kept on the same datastore. When `false`, Storage DRS may locate
* individual disks on different datastores if it helps satisfy cluster
* requirements. When not specified, the datastore cluster's settings are used.
*
*/
@Export(name="sdrsIntraVmAffinity", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sdrsIntraVmAffinity;
/**
* @return Overrides the intra-VM affinity setting
* for this virtual machine. When `true`, all disks for this virtual machine
* will be kept on the same datastore. When `false`, Storage DRS may locate
* individual disks on different datastores if it helps satisfy cluster
* requirements. When not specified, the datastore cluster's settings are used.
*
*/
public Output> sdrsIntraVmAffinity() {
return Codegen.optional(this.sdrsIntraVmAffinity);
}
/**
* The UUID of the virtual machine to create
* the override for. Forces a new resource if changed.
*
*/
@Export(name="virtualMachineId", refs={String.class}, tree="[0]")
private Output virtualMachineId;
/**
* @return The UUID of the virtual machine to create
* the override for. Forces a new resource if changed.
*
*/
public Output virtualMachineId() {
return this.virtualMachineId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public StorageDrsVmOverride(java.lang.String name) {
this(name, StorageDrsVmOverrideArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public StorageDrsVmOverride(java.lang.String name, StorageDrsVmOverrideArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public StorageDrsVmOverride(java.lang.String name, StorageDrsVmOverrideArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/storageDrsVmOverride:StorageDrsVmOverride", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private StorageDrsVmOverride(java.lang.String name, Output id, @Nullable StorageDrsVmOverrideState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("vsphere:index/storageDrsVmOverride:StorageDrsVmOverride", name, state, makeResourceOptions(options, id), false);
}
private static StorageDrsVmOverrideArgs makeArgs(StorageDrsVmOverrideArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? StorageDrsVmOverrideArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static StorageDrsVmOverride get(java.lang.String name, Output id, @Nullable StorageDrsVmOverrideState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new StorageDrsVmOverride(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy