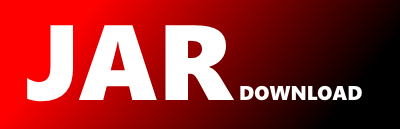
com.pulumi.vsphere.VirtualMachineSnapshotArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VirtualMachineSnapshotArgs extends com.pulumi.resources.ResourceArgs {
public static final VirtualMachineSnapshotArgs Empty = new VirtualMachineSnapshotArgs();
/**
* If set to `true`, the delta disks involved in this
* snapshot will be consolidated into the parent when this resource is
* destroyed.
*
*/
@Import(name="consolidate")
private @Nullable Output consolidate;
/**
* @return If set to `true`, the delta disks involved in this
* snapshot will be consolidated into the parent when this resource is
* destroyed.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy