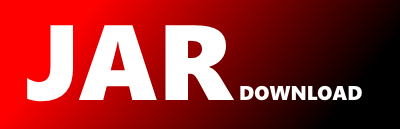
com.pulumi.vsphere.inputs.GetVirtualMachineArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.vsphere.inputs.GetVirtualMachineVappArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetVirtualMachineArgs extends com.pulumi.resources.InvokeArgs {
public static final GetVirtualMachineArgs Empty = new GetVirtualMachineArgs();
/**
* The alternate guest name of the virtual machine when
* `guest_id` is a non-specific operating system, like `otherGuest` or
* `otherGuest64`.
*
*/
@Import(name="alternateGuestName")
private @Nullable Output alternateGuestName;
/**
* @return The alternate guest name of the virtual machine when
* `guest_id` is a non-specific operating system, like `otherGuest` or
* `otherGuest64`.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy