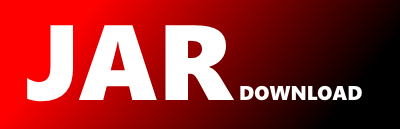
com.pulumi.vsphere.inputs.GuestOsCustomizationSpecWindowsOptionsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vsphere Show documentation
Show all versions of vsphere Show documentation
A Pulumi package for creating vsphere resources
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.vsphere.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GuestOsCustomizationSpecWindowsOptionsArgs extends com.pulumi.resources.ResourceArgs {
public static final GuestOsCustomizationSpecWindowsOptionsArgs Empty = new GuestOsCustomizationSpecWindowsOptionsArgs();
/**
* The new administrator password for this virtual machine.
*
*/
@Import(name="adminPassword")
private @Nullable Output adminPassword;
/**
* @return The new administrator password for this virtual machine.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy