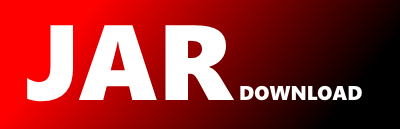
com.purej.vminspect.data.SystemData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of purej-vminspect Show documentation
Show all versions of purej-vminspect Show documentation
An easy to use, feature-rich, JMX-based and embeddable Java VM monitoring tool with a web-based user-interface
// Copyright (c), 2013, adopus consulting GmbH Switzerland, all rights reserved.
package com.purej.vminspect.data;
import java.lang.management.ClassLoadingMXBean;
import java.lang.management.GarbageCollectorMXBean;
import java.lang.management.ManagementFactory;
import java.lang.management.MemoryMXBean;
import java.lang.management.OperatingSystemMXBean;
import java.lang.management.RuntimeMXBean;
import java.lang.management.ThreadMXBean;
import java.lang.reflect.Method;
import java.net.InetAddress;
import java.util.Date;
import java.util.Map;
/**
* Provides information about the virtual machine currently running in.
*
* @author Stefan Mueller
*/
public class SystemData {
/**
* The host name and IP address.
*/
public static final String HOST;
static {
String hostName;
try {
InetAddress localHost = InetAddress.getLocalHost();
hostName = localHost.getHostName() + "@" + localHost.getHostAddress();
}
catch (Exception e) {
hostName = "127.0.0.1";
}
HOST = hostName;
}
private final String _rtInfo;
private final RuntimeMXBean _rtb;
private final OperatingSystemMXBean _osb;
private final int _threadCurrentCount;
private final int _clLoadedClassCount;
private final long _clTotalLoadedClassCount;
private final long _gcCollectionCount;
private final long _gcCollectionTimeMillis;
private final MemoryData _memoryHeap;
private final MemoryData _memoryNonHeap;
private final MemoryData _memoryPhysical;
private final MemoryData _memorySwap;
private final long _processCpuTimeMillis;
private final double _processCpuLoadPct;
private final double _systemCpuLoadPct;
/**
* Creates a new instance of this class.
*/
public SystemData() {
// Store runtime-infos:
_rtInfo = System.getProperty("java.runtime.name") + ", " + System.getProperty("java.runtime.version");
_rtb = ManagementFactory.getRuntimeMXBean();
// Store thread-infos:
ThreadMXBean tb = ManagementFactory.getThreadMXBean();
_threadCurrentCount = tb.getThreadCount();
// Get class-loader stuff:
ClassLoadingMXBean clb = ManagementFactory.getClassLoadingMXBean();
_clLoadedClassCount = clb.getLoadedClassCount();
_clTotalLoadedClassCount = clb.getTotalLoadedClassCount();
// Build gc collection time:
long tmpGcCollectionCount = 0;
long tmpGcCollectionTimeMillis = 0;
for (GarbageCollectorMXBean garbageCollector : ManagementFactory.getGarbageCollectorMXBeans()) {
tmpGcCollectionCount += garbageCollector.getCollectionCount();
tmpGcCollectionTimeMillis += garbageCollector.getCollectionTime();
}
_gcCollectionCount = tmpGcCollectionCount;
_gcCollectionTimeMillis = tmpGcCollectionTimeMillis;
// Get heap / none heap memory:
MemoryMXBean mb = ManagementFactory.getMemoryMXBean();
_memoryHeap = new MemoryData(mb.getHeapMemoryUsage());
_memoryNonHeap = new MemoryData(mb.getNonHeapMemoryUsage());
// Physical / swap memory - is hidden, type is long (eg. bytes):
_osb = ManagementFactory.getOperatingSystemMXBean();
long memPhysTotal = getHiddenInfoLong(_osb, "getTotalPhysicalMemorySize");
long memPhysFree = getHiddenInfoLong(_osb, "getFreePhysicalMemorySize");
_memoryPhysical = new MemoryData(memPhysTotal != -1 ? memPhysTotal - memPhysFree : -1, -1, memPhysTotal);
long memSwapTotal = getHiddenInfoLong(_osb, "getTotalSwapSpaceSize");
long memSwapFree = getHiddenInfoLong(_osb, "getFreeSwapSpaceSize");
_memorySwap = new MemoryData(memSwapTotal != -1 ? memSwapTotal - memSwapFree : -1, -1, memSwapTotal);
// Process Cpu time is hidden - value is in nanoseconds:
Object cpuTime = getHiddenInfo(_osb, "getProcessCpuTime");
_processCpuTimeMillis = cpuTime != null ? ((Long) cpuTime).longValue() / 1000000 : -1;
// Process Cpu load is hidden - value is a double between 0..1:
Object cpuLoad = getHiddenInfo(_osb, "getProcessCpuLoad");
double processCpuLoadPct = cpuLoad != null ? ((Double) cpuLoad).doubleValue() : -1;
_processCpuLoadPct = processCpuLoadPct < 0 ? processCpuLoadPct : processCpuLoadPct * 100;
// System Cpu load is hidden - value is a double between 0..1:
Object systemLoad = getHiddenInfo(_osb, "getSystemCpuLoad");
double systemCpuLoadPct = systemLoad != null ? ((Double) systemLoad).doubleValue() : -1;
_systemCpuLoadPct = systemCpuLoadPct < 0 ? systemCpuLoadPct : systemCpuLoadPct * 100;
}
private static long getHiddenInfoLong(Object object, String methodName) {
Object hiddenInfo = getHiddenInfo(object, methodName);
return hiddenInfo != null ? ((Long) hiddenInfo).longValue() : -1;
}
private static Object getHiddenInfo(Object object, String methodName) {
try {
Method method = object.getClass().getMethod(methodName, new Class>[0]);
method.setAccessible(true);
return method.invoke(object, new Object[0]);
}
catch (Exception e) {
// Ignore, info might not exist on this platform:
return null;
}
}
/**
* Returns the java name and version.
*/
public String getRtInfo() {
return _rtInfo;
}
/**
* The name of the runtime process.
*/
public String getRtProcessName() {
return _rtb.getName();
}
/**
* Returns the startup time of this runtime process.
*/
public Date getRtProcessStartup() {
return new Date(_rtb.getStartTime());
}
/**
* Returns the input arguments passed to the Java virtual machine.
*/
public String getRtProcessArguments() {
StringBuilder result = new StringBuilder();
for (String jvmArg : _rtb.getInputArguments()) {
if (result.length() > 0) {
result.append('\n');
}
result.append(jvmArg);
}
return result.toString();
}
/**
* Returns the current system properties.
*/
public String getRtSystemProperties() {
StringBuilder result = new StringBuilder();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy