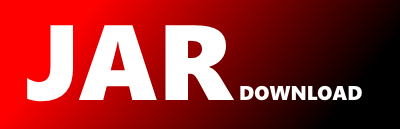
com.purej.vminspect.http.HttpResourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of purej-vminspect Show documentation
Show all versions of purej-vminspect Show documentation
An easy to use, feature-rich, JMX-based and embeddable Java VM monitoring tool with a web-based user-interface
// Copyright (c), 2013, adopus consulting GmbH Switzerland, all rights reserved.
package com.purej.vminspect.http;
import java.io.BufferedInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
/**
* Http response that reads directly from file (eg. .png, .js, .css etc.).
*
* @author Stefan Mueller
*/
public final class HttpResourceResponse extends HttpResponse {
private final String _resource;
/**
* Creates a new instance of this class.
*
* @param resource the resource
*/
public HttpResourceResponse(String resource) {
super(getContentType(resource), 14400); // 4 hour cache for resources...
_resource = resource.replace("..", ""); // For security reason!;
}
private static String getContentType(String resource) {
if (resource.endsWith(".css")) {
return "text/css";
}
else if (resource.endsWith(".js")) {
return "application/x-javascript";
}
else if (resource.endsWith(".gif")) {
return "image/gif";
}
else if (resource.endsWith(".png")) {
return "image/png";
}
else {
throw new IllegalArgumentException("Not supported resource type '" + resource + "'!");
}
}
@Override
public byte[] getContentBytes() throws IOException {
InputStream input = HttpResourceResponse.class.getResourceAsStream("/res/" + _resource);
if (input == null) {
return null;
}
try {
// Transfer from input source to byte-array:
input = new BufferedInputStream(input);
ByteArrayOutputStream out = new ByteArrayOutputStream(2048);
byte[] bytes = new byte[2048];
int length;
while ((length = input.read(bytes)) != -1) {
out.write(bytes, 0, length);
}
return out.toByteArray();
}
finally {
input.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy