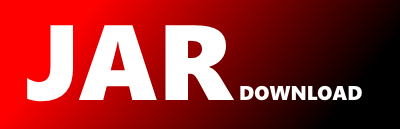
com.puresoltechnologies.graphs.trees.TreeLink Maven / Gradle / Ivy
The newest version!
package com.puresoltechnologies.graphs.trees;
import com.puresoltechnologies.graphs.graph.Edge;
import com.puresoltechnologies.graphs.graph.Pair;
public class TreeLink> implements Edge> {
private final N first;
private final N second;
public TreeLink(N first, N second) {
super();
this.first = first;
this.second = second;
}
@Override
public Pair getVertices() {
return new Pair<>(first, second);
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((first == null) ? 0 : first.hashCode());
result = prime * result + ((second == null) ? 0 : second.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
TreeLink> other = (TreeLink>) obj;
if (first == null) {
if (other.first != null)
return false;
} else if (!first.equals(other.first))
return false;
if (second == null) {
if (other.second != null)
return false;
} else if (!second.equals(other.second))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy