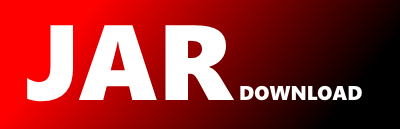
com.puresoltechnologies.purifinity.server.common.test.PerformanceTester Maven / Gradle / Ivy
package com.puresoltechnologies.purifinity.server.common.test;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
public class PerformanceTester {
public static PerformanceTestResult runPerformanceTest(
int numberOfThreads, final int actionsPerThread,
final PerformanceTest test) throws InterruptedException {
ExecutorService executor = Executors
.newFixedThreadPool(numberOfThreads);
long start = System.currentTimeMillis();
List>> futures = new ArrayList<>();
for (int threadId = 0; threadId < numberOfThreads; threadId++) {
final int finalThreadId = threadId;
Future
© 2015 - 2025 Weber Informatics LLC | Privacy Policy