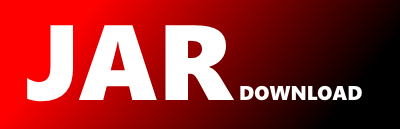
src-html.com.pusher.client.channel.Channel.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pusher-java-client Show documentation
Show all versions of pusher-java-client Show documentation
This is a Java client library for Pusher, targeted at core Java and Android.
Source code
001package com.pusher.client.channel;
002
003/**
004 * An object that represents a Pusher channel. An implementation of this
005 * interface is returned when you call
006 * {@link com.pusher.client.Pusher#subscribe(String)} or
007 * {@link com.pusher.client.Pusher#subscribe(String, ChannelEventListener, String...)}
008 * .
009 *
010 */
011public interface Channel {
012
013 /**
014 * Gets the name of the Pusher channel that this object represents.
015 *
016 * @return The name of the channel.
017 */
018 String getName();
019
020 /**
021 * Binds a {@link SubscriptionEventListener} to an event. The
022 * {@link SubscriptionEventListener} will be notified whenever the specified
023 * event is received on this channel.
024 *
025 * @param eventName
026 * The name of the event to listen to.
027 * @param listener
028 * A listener to receive notifications when the event is
029 * received.
030 * @throws IllegalArgumentException
031 * If either of the following are true:
032 * <ul>
033 * <li>The name of the event is null.</li>
034 * <li>The {@link SubscriptionEventListener} is null.</li>
035 * </ul>
036 * @throws IllegalStateException
037 * If the channel has been unsubscribed by calling
038 * {@link com.pusher.client.Pusher#unsubscribe(String)}. This
039 * puts the {@linkplain Channel} in a terminal state from which
040 * it can no longer be used. To resubscribe, call
041 * {@link com.pusher.client.Pusher#subscribe(String)} or
042 * {@link com.pusher.client.Pusher#subscribe(String, ChannelEventListener, String...)}
043 * again to receive a fresh {@linkplain Channel} instance.
044 */
045 void bind(String eventName, SubscriptionEventListener listener);
046
047 /**
048 * <p>
049 * Unbinds a previously bound {@link SubscriptionEventListener} from an
050 * event. The {@link SubscriptionEventListener} will no longer be notified
051 * whenever the specified event is received on this channel.
052 * </p>
053 *
054 * <p>
055 * Calling this method does not unsubscribe from the channel even if there
056 * are no more {@link SubscriptionEventListener}s bound to it. If you want
057 * to unsubscribe from the channel completely, call
058 * {@link com.pusher.client.Pusher#unsubscribe(String)}. It is not necessary
059 * to unbind your {@link SubscriptionEventListener}s first.
060 * </p>
061 *
062 * @param eventName
063 * The name of the event to stop listening to.
064 * @param listener
065 * The listener to unbind from the event.
066 * @throws IllegalArgumentException
067 * If either of the following are true:
068 * <ul>
069 * <li>The name of the event is null.</li>
070 * <li>The {@link SubscriptionEventListener} is null.</li>
071 * </ul>
072 * @throws IllegalStateException
073 * If the channel has been unsubscribed by calling
074 * {@link com.pusher.client.Pusher#unsubscribe(String)}. This
075 * puts the {@linkplain Channel} in a terminal state from which
076 * it can no longer be used. To resubscribe, call
077 * {@link com.pusher.client.Pusher#subscribe(String)} or
078 * {@link com.pusher.client.Pusher#subscribe(String, ChannelEventListener, String...)}
079 * again to receive a fresh {@linkplain Channel} instance.
080 */
081 void unbind(String eventName, SubscriptionEventListener listener);
082
083 /**
084 *
085 * @return Whether or not the channel is subscribed.
086 */
087 boolean isSubscribed();
088}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy