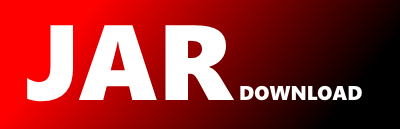
com.pusher.client.Pusher.html Maven / Gradle / Ivy
Show all versions of pusher-java-client Show documentation
Pusher (Pusher Java Websocket API)
com.pusher.client
Class Pusher
- java.lang.Object
-
- com.pusher.client.Pusher
-
- All Implemented Interfaces:
- Client
public class Pusher
extends java.lang.Object
implements Client
This class is the main entry point for accessing Pusher.
By creating a new Pusher
instance and calling connect()
a connection to Pusher is established.
Subscriptions for data are represented by
Channel
objects, or subclasses thereof.
Subscriptions are created by calling subscribe(String)
,
subscribePrivate(String)
,
subscribePresence(String)
or one of the overloads.
-
-
Constructor Summary
Constructors
Constructor and Description
Pusher(java.lang.String apiKey)
Creates a new instance of Pusher.
Pusher(java.lang.String apiKey,
PusherOptions pusherOptions)
Creates a new instance of Pusher.
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
void
connect()
Connects to Pusher.
void
connect(ConnectionEventListener eventListener,
ConnectionState... connectionStates)
Binds a ConnectionEventListener
to the specified events and then
connects to Pusher.
void
disconnect()
Disconnect from Pusher.
Channel
getChannel(java.lang.String channelName)
Connection
getConnection()
Gets the underlying Connection
object that is being used by this
instance of Pusher.
PresenceChannel
getPresenceChannel(java.lang.String channelName)
PrivateChannel
getPrivateChannel(java.lang.String channelName)
Channel
subscribe(java.lang.String channelName)
Subscribes to a public Channel
.
Channel
subscribe(java.lang.String channelName,
ChannelEventListener listener,
java.lang.String... eventNames)
Binds a ChannelEventListener
to the specified events and then
subscribes to a public Channel
.
PresenceChannel
subscribePresence(java.lang.String channelName)
Subscribes to a PresenceChannel
which
requires authentication.
PresenceChannel
subscribePresence(java.lang.String channelName,
PresenceChannelEventListener listener,
java.lang.String... eventNames)
Subscribes to a PresenceChannel
which
requires authentication.
PrivateChannel
subscribePrivate(java.lang.String channelName)
Subscribes to a PrivateChannel
which
requires authentication.
PrivateChannel
subscribePrivate(java.lang.String channelName,
PrivateChannelEventListener listener,
java.lang.String... eventNames)
Subscribes to a PrivateChannel
which
requires authentication.
void
unsubscribe(java.lang.String channelName)
Unsubscribes from a channel using via the name of the channel.
-
-
Constructor Detail
-
Pusher
public Pusher(java.lang.String apiKey)
Creates a new instance of Pusher.
Note that if you use this constructor you will not be able to subscribe
to private or presence channels because no Authorizer
has been
set. If you want to use private or presence channels:
- Create an implementation of the
Authorizer
interface, or use
the HttpAuthorizer
provided.
- Create an instance of
PusherOptions
and set the authorizer on
it by calling PusherOptions.setAuthorizer(Authorizer)
.
- Use the
Pusher(String, PusherOptions)
constructor to create
an instance of Pusher.
The PrivateChannelExampleApp
and
PresenceChannelExampleApp
example
applications show how to do this.
- Parameters:
apiKey
- Your Pusher API key.
-
Pusher
public Pusher(java.lang.String apiKey,
PusherOptions pusherOptions)
Creates a new instance of Pusher.
- Parameters:
apiKey
- Your Pusher API key.
pusherOptions
- Options for the Pusher client library to use.
-
Method Detail
-
getConnection
public Connection getConnection()
Gets the underlying Connection
object that is being used by this
instance of Pusher.
- Specified by:
getConnection
in interface Client
- Returns:
- The
Connection
object.
-
connect
public void connect()
Connects to Pusher. Any ConnectionEventListener
s that have
already been registered using the
Connection.bind(ConnectionState, ConnectionEventListener)
method
will receive connection events.
Calls are ignored (a connection is not attempted) if the Connection.getState()
is not ConnectionState.DISCONNECTED
.
-
connect
public void connect(ConnectionEventListener eventListener,
ConnectionState... connectionStates)
Binds a ConnectionEventListener
to the specified events and then
connects to Pusher. This is equivalent to binding a
ConnectionEventListener
using the
Connection.bind(ConnectionState, ConnectionEventListener)
method
before connecting.
Calls are ignored (a connection is not attempted) if the Connection.getState()
is not ConnectionState.DISCONNECTED
.
- Specified by:
connect
in interface Client
- Parameters:
eventListener
- A ConnectionEventListener
that will receive connection
events. This can be null if you are not interested in
receiving connection events, in which case you should call
connect()
instead of this method.
connectionStates
- An optional list of ConnectionState
s to bind your
ConnectionEventListener
to before connecting to
Pusher. If you do not specify any ConnectionState
s
then your ConnectionEventListener
will be bound to all
connection events. This is equivalent to calling
connect(ConnectionEventListener, ConnectionState...)
with ConnectionState.ALL
.
- Throws:
java.lang.IllegalArgumentException
- If the ConnectionEventListener
is null and at least
one connection state has been specified.
-
disconnect
public void disconnect()
Disconnect from Pusher.
Calls are ignored if the Connection.getState()
, retrieved from getConnection()
, is not
ConnectionState.CONNECTED
.
- Specified by:
disconnect
in interface Client
-
subscribe
public Channel subscribe(java.lang.String channelName)
Subscribes to a public Channel
.
Note that subscriptions should be registered only once with a Pusher
instance. Subscriptions are persisted over disconnection and
re-registered with the server automatically on reconnection. This means
that subscriptions may also be registered before connect() is called,
they will be initiated on connection.
-
subscribe
public Channel subscribe(java.lang.String channelName,
ChannelEventListener listener,
java.lang.String... eventNames)
Binds a ChannelEventListener
to the specified events and then
subscribes to a public Channel
.
- Specified by:
subscribe
in interface Client
- Parameters:
channelName
- The name of the Channel
to subscribe to.
listener
- A ChannelEventListener
to receive events. This can be
null if you don't want to bind a listener at subscription
time, in which case you should call subscribe(String)
instead of this method.
eventNames
- An optional list of event names to bind your
ChannelEventListener
to before subscribing.
- Returns:
- The
Channel
object representing your subscription.
- Throws:
java.lang.IllegalArgumentException
- If any of the following are true:
- The channel name is null.
- You are already subscribed to this channel.
- The channel name starts with "private-". If you want to
subscribe to a private channel, call
subscribePrivate(String, PrivateChannelEventListener, String...)
instead of this method.
- At least one of the specified event names is null.
- You have specified at least one event name and your
ChannelEventListener
is null.
-
subscribePrivate
public PrivateChannel subscribePrivate(java.lang.String channelName)
Subscribes to a PrivateChannel
which
requires authentication.
- Specified by:
subscribePrivate
in interface Client
- Parameters:
channelName
- The name of the channel to subscribe to.
- Returns:
- A new
PrivateChannel
representing the subscription.
- Throws:
java.lang.IllegalStateException
- if a Authorizer
has not been set
for the Pusher
instance via
Pusher(String, PusherOptions)
.
-
subscribePrivate
public PrivateChannel subscribePrivate(java.lang.String channelName,
PrivateChannelEventListener listener,
java.lang.String... eventNames)
Subscribes to a PrivateChannel
which
requires authentication.
- Specified by:
subscribePrivate
in interface Client
- Parameters:
channelName
- The name of the channel to subscribe to.
listener
- A listener to be informed of both Pusher channel protocol events and subscription data events.
eventNames
- An optional list of names of events to be bound to on the channel. The equivalent of calling Channel.bind(String, SubscriptionEventListener)
one or more times.
- Returns:
- A new
PrivateChannel
representing the subscription.
- Throws:
java.lang.IllegalStateException
- if a Authorizer
has not been set for the Pusher
instance via Pusher(String, PusherOptions)
.
-
subscribePresence
public PresenceChannel subscribePresence(java.lang.String channelName)
Subscribes to a PresenceChannel
which
requires authentication.
- Specified by:
subscribePresence
in interface Client
- Parameters:
channelName
- The name of the channel to subscribe to.
- Returns:
- A new
PresenceChannel
representing the subscription.
- Throws:
java.lang.IllegalStateException
- if a Authorizer
has not been set
for the Pusher
instance via
Pusher(String, PusherOptions)
.
-
subscribePresence
public PresenceChannel subscribePresence(java.lang.String channelName,
PresenceChannelEventListener listener,
java.lang.String... eventNames)
Subscribes to a PresenceChannel
which
requires authentication.
- Specified by:
subscribePresence
in interface Client
- Parameters:
channelName
- The name of the channel to subscribe to.
listener
- A listener to be informed of Pusher channel protocol, including presence-specific events, and subscription data events.
eventNames
- An optional list of names of events to be bound to on the channel. The equivalent of calling Channel.bind(String, SubscriptionEventListener)
one or more times.
- Returns:
- A new
PresenceChannel
representing the subscription.
- Throws:
java.lang.IllegalStateException
- if a Authorizer
has not been set for the Pusher
instance via Pusher(String, PusherOptions)
.
-
unsubscribe
public void unsubscribe(java.lang.String channelName)
Unsubscribes from a channel using via the name of the channel.
- Specified by:
unsubscribe
in interface Client
- Parameters:
channelName
- the name of the channel to be unsubscribed from.
-
getChannel
public Channel getChannel(java.lang.String channelName)
- Specified by:
getChannel
in interface Client
- Parameters:
channelName
- The name of the public channel to be retrieved
- Returns:
- A public channel, or null if it could not be found
- Throws:
java.lang.IllegalArgumentException
- if you try to retrieve a private or presence channel.
-
getPrivateChannel
public PrivateChannel getPrivateChannel(java.lang.String channelName)
- Specified by:
getPrivateChannel
in interface Client
- Parameters:
channelName
- The name of the private channel to be retrieved
- Returns:
- A private channel, or null if it could not be found
- Throws:
java.lang.IllegalArgumentException
- if you try to retrieve a public or presence channel.
-
getPresenceChannel
public PresenceChannel getPresenceChannel(java.lang.String channelName)
- Specified by:
getPresenceChannel
in interface Client
- Parameters:
channelName
- The name of the presence channel to be retrieved
- Returns:
- A presence channel, or null if it could not be found
- Throws:
java.lang.IllegalArgumentException
- if you try to retrieve a public or private channel.