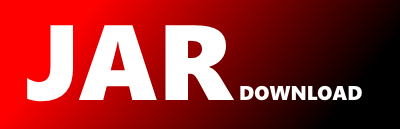
src-html.com.pusher.client.channel.User.html Maven / Gradle / Ivy
Source code
001package com.pusher.client.channel;
002
003import com.google.gson.Gson;
004
005/**
006 * Represents a user that is subscribed to a
007 * {@link com.pusher.client.channel.PresenceChannel PresenceChannel}.
008 */
009public class User {
010 private static final Gson GSON = new Gson();
011 private final String id;
012 private final String jsonData;
013
014 /**
015 * Create a new user. Users should not be created within an application.
016 * Users are created within the library and represent subscriptions to
017 * presence channels.
018 *
019 * @param id The user id
020 * @param jsonData The user JSON data
021 */
022 public User(final String id, final String jsonData) {
023 this.id = id;
024 this.jsonData = jsonData;
025 }
026
027 /**
028 * A unique identifier for the user within a Pusher application.
029 *
030 * @return The unique id.
031 */
032 public String getId() {
033 return id;
034 }
035
036 /**
037 * Custom additional information about a user as a String encoding a JSON
038 * hash
039 *
040 * @return The user info as a JSON string
041 */
042 public String getInfo() {
043 return jsonData;
044 }
045
046 /**
047 * <p>
048 * Custom additional information about a user decoded as a new instance of
049 * the provided POJO bean type
050 * </p>
051 *
052 * <p>
053 * e.g. if {@link #getInfo()} returns
054 * <code>{"name":"Mr User","number":9}</code> then you might implement as
055 * follows:
056 * </p>
057 *
058 * <pre>
059 * public class UserInfo {
060 * private String name;
061 * private Integer number;
062 *
063 * public String getName() { return name; }
064 * public void setName(String name) { this.name = name; }
065 *
066 * public Integer getNumber() { return number; }
067 * public void setNumber(Integer number) { this.number = number; }
068 * }
069 *
070 * UserInfo info = user.getInfo(UserInfo.class);
071 *
072 * info.getName() // returns "Mr User"
073 * info.getNumber() // returns 9
074 * </pre>
075 *
076 * @param <V> The class of the info
077 * @param clazz
078 * the class into which the user info JSON representation should
079 * be parsed.
080 * @return V An instance of clazz, populated with the user info
081 */
082 public <V> V getInfo(final Class<V> clazz) {
083 return GSON.fromJson(jsonData, clazz);
084 }
085
086 @Override
087 public String toString() {
088 return String.format("[User id=%s, data=%s]", id, jsonData);
089 }
090
091 @Override
092 public int hashCode() {
093 return id.hashCode() + (jsonData != null ? jsonData.hashCode() : 0);
094 }
095
096 @Override
097 public boolean equals(final Object other) {
098
099 if (other instanceof User) {
100 final User otherUser = (User)other;
101 return getId().equals(otherUser.getId()) && this.getInfo().equals(otherUser.getInfo());
102 }
103
104 return false;
105 }
106}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy