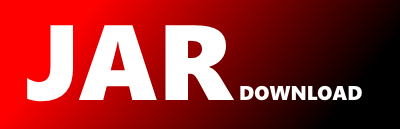
src-html.com.pusher.client.util.Factory.html Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pusher-java-client Show documentation
Show all versions of pusher-java-client Show documentation
This is a Java client library for Pusher, targeted at core Java and Android.
Source code
001package com.pusher.client.util;
002
003import java.net.Proxy;
004import java.net.URI;
005import java.net.URISyntaxException;
006import java.util.concurrent.ExecutorService;
007import java.util.concurrent.Executors;
008import java.util.concurrent.ScheduledExecutorService;
009import java.util.concurrent.ThreadFactory;
010
011import javax.net.ssl.SSLException;
012
013import com.pusher.client.Authorizer;
014import com.pusher.client.PusherOptions;
015import com.pusher.client.channel.impl.ChannelImpl;
016import com.pusher.client.channel.impl.ChannelManager;
017import com.pusher.client.channel.impl.PresenceChannelImpl;
018import com.pusher.client.channel.impl.PrivateChannelImpl;
019import com.pusher.client.connection.impl.InternalConnection;
020import com.pusher.client.connection.websocket.WebSocketClientWrapper;
021import com.pusher.client.connection.websocket.WebSocketConnection;
022import com.pusher.client.connection.websocket.WebSocketListener;
023
024/**
025 * This is a lightweight way of doing dependency injection and enabling classes
026 * to be unit tested in isolation. No class in this library instantiates another
027 * class directly, otherwise they would be tightly coupled. Instead, they all
028 * call the factory methods in this class when they want to create instances of
029 * another class.
030 *
031 * An instance of Factory is provided on construction to each class which may
032 * require it, the initial factory is instantiated in the Pusher constructor,
033 * the only constructor which a library consumer should need to call directly.
034 *
035 * Conventions:
036 *
037 * - any method that starts with "new", such as
038 * {@link #newPublicChannel(String)} creates a new instance of that class every
039 * time it is called.
040 *
041 */
042public class Factory {
043
044 private InternalConnection connection;
045 private ChannelManager channelManager;
046 private ExecutorService eventQueue;
047 private ScheduledExecutorService timers;
048 private static final Object eventLock = new Object();
049
050 public synchronized InternalConnection getConnection(final String apiKey, final PusherOptions options) {
051 if (connection == null) {
052 try {
053 connection = new WebSocketConnection(options.buildUrl(apiKey), options.getActivityTimeout(),
054 options.getPongTimeout(), options.getProxy(), this);
055 }
056 catch (final URISyntaxException e) {
057 throw new IllegalArgumentException("Failed to initialise connection", e);
058 }
059 }
060 return connection;
061 }
062
063 public WebSocketClientWrapper newWebSocketClientWrapper(final URI uri, final Proxy proxy, final WebSocketListener webSocketListener) throws SSLException {
064 return new WebSocketClientWrapper(uri, proxy, webSocketListener);
065 }
066
067 public synchronized ScheduledExecutorService getTimers() {
068 if (timers == null) {
069 timers = Executors.newSingleThreadScheduledExecutor(new DaemonThreadFactory("timers"));
070 }
071 return timers;
072 }
073
074 public ChannelImpl newPublicChannel(final String channelName) {
075 return new ChannelImpl(channelName, this);
076 }
077
078 public PrivateChannelImpl newPrivateChannel(final InternalConnection connection, final String channelName,
079 final Authorizer authorizer) {
080 return new PrivateChannelImpl(connection, channelName, authorizer, this);
081 }
082
083 public PresenceChannelImpl newPresenceChannel(final InternalConnection connection, final String channelName,
084 final Authorizer authorizer) {
085 return new PresenceChannelImpl(connection, channelName, authorizer, this);
086 }
087
088 public synchronized ChannelManager getChannelManager() {
089 if (channelManager == null) {
090 channelManager = new ChannelManager(this);
091 }
092 return channelManager;
093 }
094
095 public synchronized void queueOnEventThread(final Runnable r) {
096 if (eventQueue == null) {
097 eventQueue = Executors.newSingleThreadExecutor(new DaemonThreadFactory("eventQueue"));
098 }
099 eventQueue.execute(new Runnable() {
100 @Override
101 public void run() {
102 synchronized (eventLock) {
103 r.run();
104 }
105 }
106 });
107 }
108
109 public synchronized void shutdownThreads() {
110 if (eventQueue != null) {
111 eventQueue.shutdown();
112 eventQueue = null;
113 }
114 if (timers != null) {
115 timers.shutdown();
116 timers = null;
117 }
118 }
119
120 private static class DaemonThreadFactory implements ThreadFactory {
121 private final String name;
122
123 public DaemonThreadFactory(final String name) {
124 this.name = name;
125 }
126
127 @Override
128 public Thread newThread(final Runnable r) {
129 final Thread t = new Thread(r);
130 t.setDaemon(true);
131 t.setName("pusher-java-client " + name);
132 return t;
133 }
134 }
135}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy