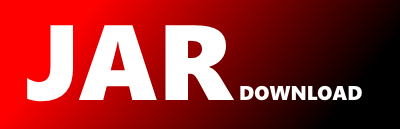
com.pusher.client.channel.impl.ChannelImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pusher-java-client Show documentation
Show all versions of pusher-java-client Show documentation
This is a Java client library for Pusher, targeted at core Java and Android.
package com.pusher.client.channel.impl;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.pusher.client.channel.*;
import com.pusher.client.util.Factory;
public class ChannelImpl implements InternalChannel {
private final Gson GSON;
private static final String INTERNAL_EVENT_PREFIX = "pusher_internal:";
protected static final String SUBSCRIPTION_SUCCESS_EVENT = "pusher_internal:subscription_succeeded";
protected final String name;
private final Map> eventNameToListenerMap = new HashMap>();
protected volatile ChannelState state = ChannelState.INITIAL;
private ChannelEventListener eventListener;
private final Factory factory;
private final Object lock = new Object();
public ChannelImpl(final String channelName, final Factory factory) {
GsonBuilder gsonBldr = new GsonBuilder();
gsonBldr.registerTypeAdapter(PusherEvent.class, new PusherEventDeserializer());
GSON = gsonBldr.create();
if (channelName == null) {
throw new IllegalArgumentException("Cannot subscribe to a channel with a null name");
}
for (final String disallowedPattern : getDisallowedNameExpressions()) {
if (channelName.matches(disallowedPattern)) {
throw new IllegalArgumentException(
"Channel name "
+ channelName
+ " is invalid. Private channel names must start with \"private-\" and presence channel names must start with \"presence-\"");
}
}
name = channelName;
this.factory = factory;
}
/* Channel implementation */
@Override
public String getName() {
return name;
}
@Override
public void bind(final String eventName, final SubscriptionEventListener listener) {
validateArguments(eventName, listener);
synchronized (lock) {
Set listeners = eventNameToListenerMap.get(eventName);
if (listeners == null) {
listeners = new HashSet();
eventNameToListenerMap.put(eventName, listeners);
}
listeners.add(listener);
}
}
@Override
public void unbind(final String eventName, final SubscriptionEventListener listener) {
validateArguments(eventName, listener);
synchronized (lock) {
final Set listeners = eventNameToListenerMap.get(eventName);
if (listeners != null) {
listeners.remove(listener);
if (listeners.isEmpty()) {
eventNameToListenerMap.remove(eventName);
}
}
}
}
@Override
public boolean isSubscribed() {
return state == ChannelState.SUBSCRIBED;
}
/* InternalChannel implementation */
@Override
public void onMessage(final String event, final String message) {
if (event.equals(SUBSCRIPTION_SUCCESS_EVENT)) {
updateState(ChannelState.SUBSCRIBED);
}
else {
final Set listeners;
synchronized (lock) {
final Set sharedListeners = eventNameToListenerMap.get(event);
if (sharedListeners != null) {
listeners = new HashSet(sharedListeners);
}
else {
listeners = null;
}
}
if (listeners != null) {
for (final SubscriptionEventListener listener : listeners) {
final PusherEvent e = GSON.fromJson(message, PusherEvent.class);
factory.queueOnEventThread(new Runnable() {
@Override
public void run() {
listener.onEvent(e);
}
});
}
}
}
}
@Override
public String toSubscribeMessage() {
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy