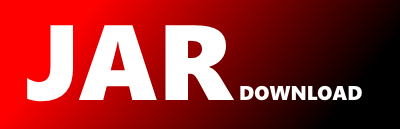
com.pyx4j.jni.Beep Maven / Gradle / Ivy
/*
* Pyx4j framework
* Copyright (C) 2006 pyx4.com.
*
* @author vlads
* @version $Id: Beep.java 1 2007-03-09 04:03:48Z vlads $
*/
package com.pyx4j.jni;
import java.util.List;
import java.util.StringTokenizer;
import java.util.Vector;
/**
* Genrate beep on windows
*/
public class Beep {
static {
Common.isAvailable();
}
/**
*
* @param freq The frequency of the sound, in hertz. This parameter must be in the range 37 through 32,767
* @param duration The duration of the sound, in milliseconds.
*/
public static native void beep(int freq, int duration);
public static Thread sound(final int[] args) {
if (!Common.isAvailable()) {
return null;
}
Runnable async = new Runnable() {
public void run() {
int repeate_count = 0;
for (int i = 0; i < (args.length - 1); i += 2) {
if (args[i] < 0) {
// Repeate
int cnt = -args[i];
if (repeate_count > cnt) {
break;
}
repeate_count ++;
i = 0;
try {
Thread.sleep(args[i + 1]);
} catch (InterruptedException e) {
break;
}
} else if (args[i] == 0) {
try {
Thread.sleep(args[i + 1]);
} catch (InterruptedException e) {
break;
}
} else {
beep(args[i], args[i + 1]);
}
}
}
};
Thread t = new Thread(async, "Sound");
t.setDaemon(true);
t.start();
return t;
}
public static Thread sound(String args) {
if ((args == null) || (args.length() == 0)) {
return null;
}
StringTokenizer st = new StringTokenizer(args, " ");
List ints = new Vector();
while (st.hasMoreTokens()) {
ints.add(Integer.valueOf((st.nextToken())));
}
int[] fd = new int[ints.size()];
for (int i = 0; i < ints.size(); i++) {
fd[i] = ints.get(i).intValue();
}
return sound(fd);
}
public static void main(String[] args) {
if (!Common.isAvailable()) {
System.err.println("Native DLL not avalable");
}
Thread t = null;
if (args.length < 2) {
t = sound("1500 200 0 200 2500 200 0 100 2000 200");
System.out.println("Beep $freq $duration [$freq $duration]*");
System.out.println("0 $freq is pause");
System.out.println("negative $freq is repeat count");
} else if (args.length == 2) {
beep(Integer.valueOf(args[0]).intValue(), Integer.valueOf(args[1]).intValue());
} else {
int[] fd = new int[args.length];
for (int i = 0; i < args.length; i++) {
fd[i] = Integer.valueOf(args[i]).intValue();
}
t = sound(fd);
}
while ((t != null) && (t.isAlive())) {
try {
Thread.sleep(500);
} catch (InterruptedException e) {
break;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy